- Published on
Spread types may only be created from object types in TypeScript
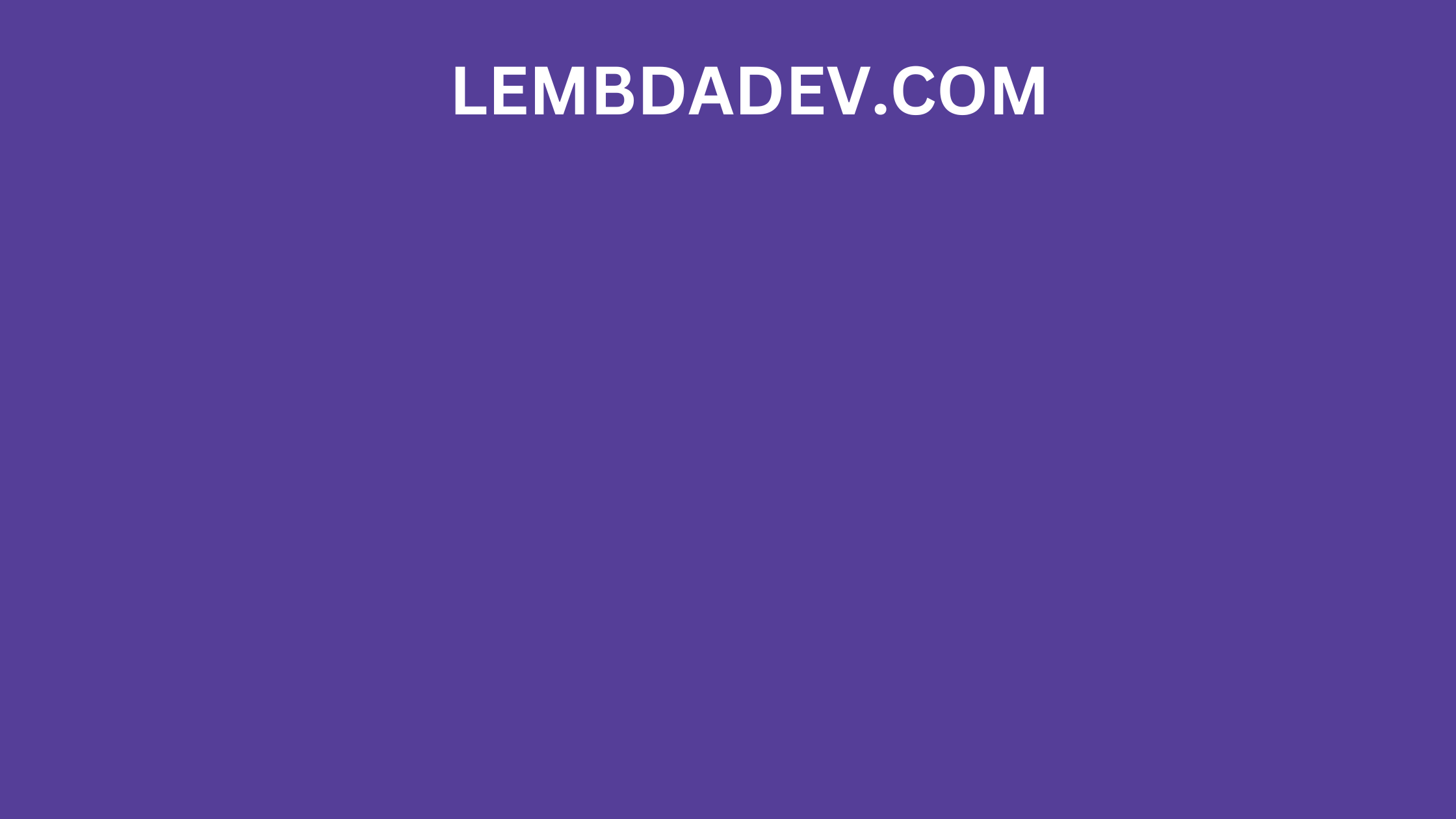
Spread types may only be created from object types in TypeScript
How to fix the "Spread types may only be created from object types in TypeScript" error
TypeScript is a typed superset of JavaScript that adds optional static type checking to the language. This means that TypeScript can help you catch errors in your code before you even run it. One of the benefits of using TypeScript is that it can help you ensure that your code is type-safe.
However, TypeScript can sometimes throw errors that you don't understand. One common error is the Spread types may only be created from object types error. This error occurs when you try to use the spread operator (...
) with a value that is not an object.
There are a few ways to fix the Spread types may only be created from object types in TypeScript error:
- Make sure that the value you are using the spread operator with is an object. This means that the value must have at least one property. For example, the following code is valid:
const object = { name: "John Doe" };
const spreadObject = { ...object };
However, the following code is not valid:
const number = 10;
const spreadNumber = { ...number }; // This will cause the error
This is because the number
variable is not an object. It is a primitive type.
- Use a type assertion to tell TypeScript that the value you are using the spread operator with is an object. This can be done using the
as
keyword. For example, the following code is valid:
const number = 10;
const spreadNumber = { ...(number as any) }; // This is valid, but not recommended
However, using a type assertion is not recommended. It is better to make sure that the value you are using the spread operator with is an object to avoid errors.
- Use a conditional statement to check if the value you are using the spread operator with is an object. If it is, then you can use the spread operator. Otherwise, you can use a different value. For example, the following code is valid:
const number = 10;
const spreadNumber = typeof number === "object" ? { ...number } : 0;
This code will check if the number
variable is an object. If it is, then the code will use the spread operator to create a new object from the number
variable. Otherwise, the code will assign the value 0 to the spreadNumber
variable.
I hope this helps!
Related Artcles
- Type useState as Array or Object in React TypeScript
- Type the onFocus and onBlur events in React TypeScript
- Type onKeyDown, onKeyUp, onKeyPress events in React(TypeScript)
- Type the onClick event of an element in React(TypeScript)
- Type the onChange event of an element in React(TypeScript)
- Type the onSubmit event in React (TypeScript)
- How to type a Date object in TypeScript
- Define types for process.env in TypeScript
- How to type an async Function in TypeScript
- How to Type the reduce() method in TypeScript