- Published on
How to Type useState as Array or Object in React TypeScript?
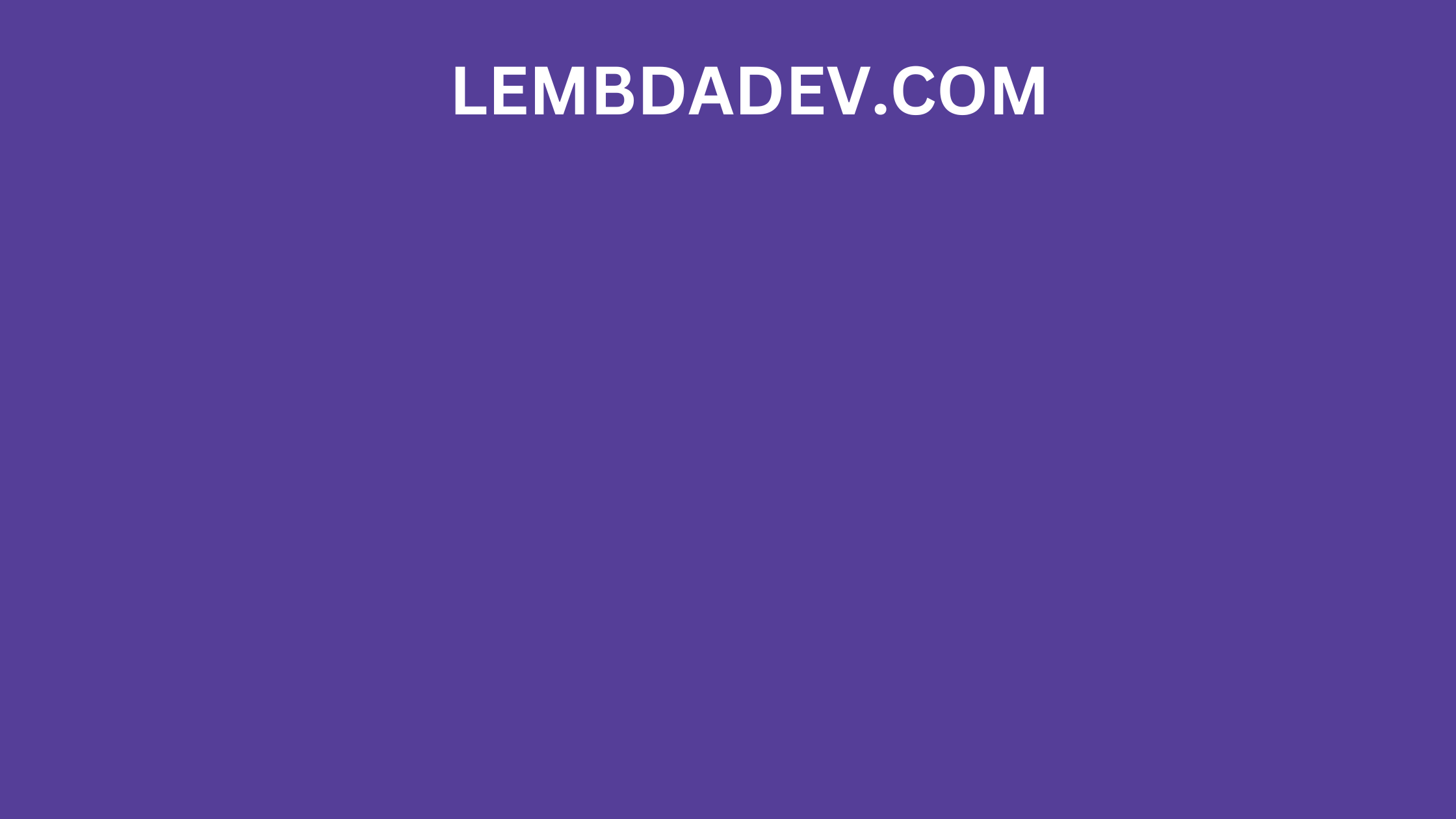
How to Type useState as Array or Object in React TypeScript?
How to Type useState as Array or Object in React TypeScript?
TypeScript is a typed superset of JavaScript that adds optional static type checking to the language. This means that TypeScript can help you catch errors in your code before you even run it. One of the benefits of using TypeScript is that it can help you type your state variables. This can make your code more readable and maintainable.
👉 👉 React Hooks Complete Guide
What is the useState hook?
The useState hook is a React hook that allows you to manage state in your React components. The useState hook returns an array with two elements: the current state value and a function to update the state value.
How to type useState as an array in React TypeScript
To type useState as an array in React TypeScript, you need to specify the type of the array. For example, the following code types useState as an array of strings:
import { useState } from "react";
const App: React.FC<{}> = () => {
const [names, setNames] = useState<string[]>([]);
return (
<div>
<ul>
{names.map((name) => (
<li key={name}>{name}</li>
))}
</ul>
<button onClick={() => setNames([...names, "John Doe"])}>Add name</button>
</div>
);
};
export default App;
The useState()
hook returns an array with two elements: the names
state variable and the setNames()
function to update the state variable. The names
state variable is typed as an array of strings, which means that it can only contain strings.
How to type useState as an object in React TypeScript
To type useState as an object in React TypeScript, you need to specify the type of the object. For example, the following code types useState as an object with a name
property and an age
property:
import { useState } from "react";
interface User {
name: string;
age: number;
}
const App: React.FC<{}> = () => {
const [user, setUser] = useState<User>({ name: "", age: 0 });
return (
<div>
<p>Name: {user.name}</p>
<p>Age: {user.age}</p>
<button onClick={() => setUser({ ...user, name: "John Doe" })}>
Update name
</button>
</div>
);
};
export default App;
The useState()
hook returns an array with two elements: the user
state variable and the setUser()
function to update the state variable. The user
state variable is typed as an object with a name
property and an age
property, which means that it can only contain objects with those properties.
Benefits of typing useState as an array or object
Typing useState as an array or object has several benefits:
- It makes your code more readable and maintainable.
- It helps you catch errors in your code before you even run it.
- It can help you prevent performance problems.
Related Artcles
- Type the onFocus and onBlur events in React TypeScript
- Type onKeyDown, onKeyUp, onKeyPress events in React(TypeScript)
- Type the onClick event of an element in React(TypeScript)
- Type the onChange event of an element in React(TypeScript)
- Type the onSubmit event in React (TypeScript)
- How to type a Date object in TypeScript
- Define types for process.env in TypeScript
- How to type an async Function in TypeScript
- How to Type the reduce() method in TypeScript
- How to Type the Spread method in TypeScript