- Published on
Comprehensive Guide to JavaScript Design Patterns
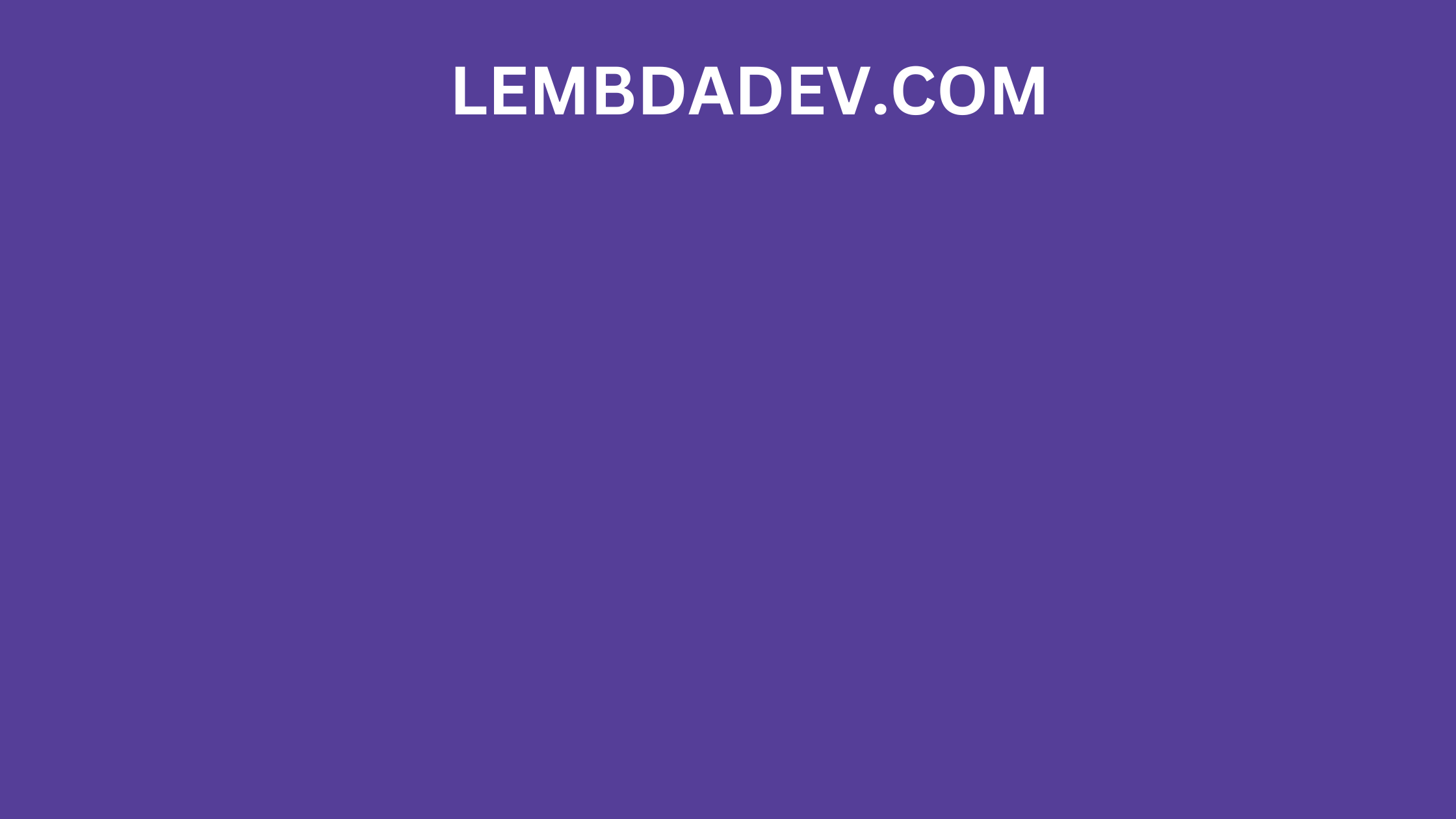
Comprehensive Guide to JavaScript Design Patterns
At work, we recognize the paramount importance of JavaScript design patterns in today's rapidly evolving web development landscape. Mastering these patterns can significantly enhance your coding practices, leading to the creation of more efficient, scalable, and maintainable applications. In this comprehensive guide, we will take a deep dive into various JavaScript design patterns, elucidating each one with detailed examples to ensure a comprehensive understanding and empower you to outshine your competitors in the digital realm.
1. Introduction
Welcome to our Comprehensive Guide to JavaScript Design Patterns. In this guide, we'll delve into the world of design patterns, specifically tailored for JavaScript development. By mastering these patterns, you'll be equipped with the tools to elevate your code quality and efficiency, giving you a competitive edge in the dynamic realm of web development.
2. Understanding Design Patterns
Design patterns are proven solutions to recurring problems in software design. They provide a structured approach to addressing common coding challenges. In the realm of JavaScript, these patterns offer a roadmap to organize your codebase and facilitate best practices, ultimately leading to more maintainable projects.
3. Why JavaScript Design Patterns Matter
The significance of JavaScript design patterns cannot be overstated. They offer a range of benefits, including enhanced code readability, streamlined codebase organization, and simplified code maintenance. By adopting these patterns, developers can capitalize on established methodologies, expediting development and ensuring optimal outcomes.
4. Creational Design Patterns
Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. This is invaluable when you need to control a resource's access or manage configurations centrally. Let's consider an example:
class Logger {
constructor() {
this.logs = [];
}
log(message) {
this.logs.push(message);
}
displayLogs() {
console.log(this.logs);
}
}
const loggerInstance = new Logger();
loggerInstance.log("Log message 1");
loggerInstance.log("Log message 2");
loggerInstance.displayLogs(); // Output: ["Log message 1", "Log message 2"]
Factory Pattern
The Factory pattern centralizes object creation, abstracting away the specifics of object instantiation. This enhances flexibility and maintains loose coupling. Let's illustrate with an example:
class Car {
constructor(make, model) {
this.make = make;
this.model = model;
}
displayInfo() {
console.log(`Make: ${this.make}, Model: ${this.model}`);
}
}
class CarFactory {
createCar(make, model) {
return new Car(make, model);
}
}
const factory = new CarFactory();
const car = factory.createCar("Toyota", "Camry");
car.displayInfo(); // Output: Make: Toyota, Model: Camry
Abstract Factory Pattern
The Abstract Factory pattern provides an interface for creating families of related or dependent objects. It ensures that objects created adhere to a common theme. Let's delve into an example:
class Shape {
draw() {
throw new Error("Abstract method 'draw' must be overridden");
}
}
class Circle extends Shape {
draw() {
console.log("Drawing a circle");
}
}
class Square extends Shape {
draw() {
console.log("Drawing a square");
}
}
class ShapeFactory {
createShape(type) {
switch (type) {
case "circle":
return new Circle();
case "square":
return new Square();
default:
throw new Error("Invalid shape type");
}
}
}
const factory = new ShapeFactory();
const circle = factory.createShape("circle");
const square = factory.createShape("square");
circle.draw(); // Output: Drawing a circle
square.draw(); // Output: Drawing a square
Builder Pattern
The Builder pattern simplifies the creation of complex objects by separating the construction process from the object's representation. This enables the creation of different representations using the same construction process. Let's exemplify:
class Burger {
constructor() {
this.size = null;
this.cheese = false;
this.pepperoni = false;
this.lettuce = false;
}
addCheese() {
this.cheese = true;
return this;
}
addPepperoni() {
this.pepperoni = true;
return this;
}
addLettuce() {
this.lettuce = true;
return this;
}
setSize(size) {
this.size = size;
return this;
}
build() {
return this;
}
}
const burger = new Burger().setSize("large").addCheese().addPepperoni().build();
console.log(burger);
// Output: Burger { size: 'large', cheese: true, pepperoni: true, lettuce: false }
5. Structural Design Patterns
Adapter Pattern
The Adapter pattern bridges the gap between incompatible interfaces, enabling collaboration between objects with differing interfaces. It's like a language translator for objects. Let's elucidate:
class CelsiusTemperature {
constructor(value) {
this.value = value;
}
getValue() {
return this.value;
}
}
class FahrenheitTemperature {
constructor(value) {
this.value = value;
}
getFahrenheitValue() {
return this.value;
}
}
class TemperatureAdapter {
constructor(temperature) {
this.temperature = temperature;
}
getValue() {
return ((this.temperature.getFahrenheitValue() - 32) * 5) / 9;
}
}
const celsiusTemp = new CelsiusTemperature(20);
const fahrenheitTemp = new FahrenheitTemperature(68);
const adaptedTemp = new TemperatureAdapter(fahrenheitTemp);
console.log(`Celsius Temperature: ${celsiusTemp.getValue()}`);
console.log(`Adapted Temperature: ${adaptedTemp.getValue()}`);
// Output: Celsius Temperature: 20
// Adapted Temperature: 20
Decorator Pattern
The Decorator pattern enhances object functionality dynamically by adding responsibilities. It's like adding layers of functionality to an object. Let's illustrate:
class Coffee {
cost() {
return 5;
}
getDescription() {
return "Coffee";
}
}
class MilkDecorator {
constructor(coffee) {
this.coffee = coffee;
}
cost() {
return this.coffee.cost() + 2;
}
getDescription() {
return `${this.coffee.getDescription()} + Milk`;
}
}
class SugarDecorator {
constructor(coffee) {
this.coffee = coffee;
}
cost() {
return this.coffee.cost() + 1;
}
getDescription() {
return `${this.coffee.getDescription()} + Sugar`;
}
}
let myCoffee = new Coffee();
myCoffee = new MilkDecorator(myCoffee);
myCoffee = new SugarDecorator(myCoffee);
console.log(`Cost: $${myCoffee.cost()}`);
console.log(`Description: ${myCoffee.getDescription()}`);
// Output: Cost: $8
// Description: Coffee + Milk + Sugar
Facade Pattern
The Facade pattern provides a simplified interface to a complex subsystem, making it easier to interact with. It's like a concierge service for your code. Let's exemplify:
class Engine {
start() {
console.log("Engine started");
}
stop() {
console.log("Engine stopped");
}
}
class Ignition {
ignite() {
console.log("Ignition ignited");
}
cutOff() {
console.log("Ignition cut off");
}
}
class Facade {
constructor(engine, ignition) {
this.engine = engine;
this.ignition = ignition;
}
startCar() {
this.engine.start();
this.ignition.ignite();
console.log("Car started");
}
stopCar() {
this.ignition.cutOff();
this.engine.stop();
console.log("Car stopped");
}
}
const engine = new Engine();
const ignition = new Ignition();
const carFacade = new Facade(engine, ignition);
carFacade.startCar(); // Output: Engine started, Ignition ignited, Car started
carFacade.stopCar(); // Output: Ignition cut off, Engine stopped, Car stopped
Composite Pattern
The Composite pattern arranges objects into tree structures to represent part-whole hierarchies. It enables treating individual objects and compositions uniformly. It's like creating a nested hierarchy of components. Let's dive into an example:
class Department {
constructor(name) {
this.name = name;
this.subDepartments = [];
}
addSubDepartment(subDepartment) {
this.subDepartments.push(subDepartment);
}
getSubDepartments() {
return this.subDepartments;
}
display() {
console.log(`Department: ${this.name}`);
}
}
const salesDepartment = new Department("Sales");
const marketingDepartment = new Department("Marketing");
const developmentDepartment = new Department("Development");
salesDepartment.addSubDepartment(marketingDepartment);
salesDepartment.addSubDepartment(developmentDepartment);
const subDepartments = salesDepartment.getSubDepartments();
for (const department of subDepartments) {
department.display();
}
// Output: Department: Marketing
// Department: Development
6. Behavioral Design Patterns
Observer Pattern
The Observer pattern establishes a dependency between objects, ensuring that when one object changes state, its dependents are notified and updated automatically. Think of it as subscribing to updates. Let's illustrate:
class Publisher {
constructor() {
this.subscribers = [];
}
subscribe(subscriber) {
this.subscribers.push(subscriber);
}
unsubscribe(subscriber) {
this.subscribers = this.subscribers.filter((sub) => sub !== subscriber);
}
notify(message) {
this.subscribers.forEach((subscriber) => subscriber.update(message));
}
}
class Subscriber {
constructor(name) {
this.name = name;
}
update(message) {
console.log(`${this.name} received message: ${message}`);
}
}
const publisher = new Publisher();
const subscriber1 = new Subscriber("Subscriber 1");
const subscriber2 = new Subscriber("Subscriber 2");
publisher.subscribe(subscriber1);
publisher.subscribe(subscriber2);
publisher.notify("New update!"); // Output: Subscriber 1 received message: New update!
// Subscriber 2 received message: New update!
Strategy Pattern
The Strategy pattern defines a family of interchangeable algorithms, allowing clients to choose from different strategies based on their needs. It's like having multiple tools in your toolkit. Let's exemplify:
class PaymentStrategy {
pay(amount) {
throw new Error("Abstract method 'pay' must be overridden");
}
}
class CreditCardPayment extends PaymentStrategy {
pay(amount) {
console.log(`Paid $${amount} using credit card`);
}
}
class PayPalPayment extends PaymentStrategy {
pay(amount) {
console.log(`Paid $${amount} using PayPal`);
}
}
class ShoppingCart {
constructor(paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
checkout(amount) {
this.paymentStrategy.pay(amount);
}
}
const creditCardPayment = new CreditCardPayment();
const payPalPayment = new PayPalPayment();
const cart1 = new ShoppingCart(creditCardPayment);
cart1.checkout(100); // Output: Paid $100 using credit card
const cart2 = new ShoppingCart(payPalPayment);
cart2.checkout(75); // Output: Paid $75 using PayPal
Command Pattern
The Command pattern encapsulates requests as objects, allowing for parameterization of clients with different requests, queuing of requests, and logging of their execution. It's like creating a to-do list for your code. Let's delve into an example:
class Light {
turnOn() {
console.log("Light is on");
}
turnOff() {
console.log("Light is off");
}
}
class LightOnCommand {
constructor(light) {
this.light = light;
}
execute() {
this.light.turnOn();
}
}
class LightOffCommand {
constructor(light) {
this.light = light;
}
execute() {
this.light.turnOff();
}
}
class RemoteControl {
constructor() {
this.command = null;
}
setCommand(command) {
this.command = command;
}
pressButton() {
this.command.execute();
}
}
const light = new Light();
const lightOnCommand = new LightOnCommand(light);
const lightOffCommand = new LightOffCommand(light);
const remoteControl = new RemoteControl();
remoteControl.setCommand(lightOnCommand);
remoteControl.pressButton(); // Output: Light is on
remoteControl.setCommand(lightOffCommand);
remoteControl.pressButton(); // Output: Light is off
Iterator Pattern
The Iterator pattern provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation. It simplifies traversal of collections. It's like paging through a book. Let's exemplify:
class Book {
constructor(title, author) {
this.title = title;
this.author = author;
}
}
class Library {
constructor() {
this.books = [];
}
addBook(book) {
this.books.push(book);
}
createIterator() {
return new LibraryIterator(this.books);
}
}
class LibraryIterator {
constructor(books) {
this.books = books;
this.currentIndex = 0;
}
hasNext() {
return this.currentIndex < this.books.length;
}
next() {
const book = this.books[this.currentIndex];
this.currentIndex++;
return book;
}
}
const library = new Library();
library.addBook(new Book("The Great Gatsby", "F. Scott Fitzgerald"));
library.addBook(new Book("To Kill a Mockingbird", "Harper Lee"));
library.addBook(new Book("1984", "George Orwell"));
const iterator = library.createIterator();
while (iterator.hasNext()) {
const book = iterator.next();
console.log(`Title: ${book.title}, Author: ${book.author}`);
}
// Output: Title: The Great Gatsby, Author: F. Scott Fitzgerald
// Title: To Kill a Mockingbird, Author: Harper Lee
// Title: 1984, Author: George Orwell
7. Implementing Design Patterns
Implementing design patterns entails adhering to best practices, leveraging real-world examples, and avoiding common pitfalls.
Best Practices and Guidelines
- Understand the problem before selecting a design pattern.
- Prioritize code readability and maintainability.
- Document the rationale behind your pattern choice.
- Continuously refactor and optimize your codebase.
Real-world Examples and Use Cases
Here are practical instances of design pattern implementation.
Implementing the Singleton Pattern
class ConfigurationManager {
constructor() {
this.config = null;
}
static getInstance() {
if (!this.instance) {
this.instance = new ConfigurationManager();
}
return this.instance;
}
loadConfig(config) {
this.config = config;
}
getConfig() {
return this.config;
}
}
const configManager = ConfigurationManager.getInstance();
configManager.loadConfig({ theme: "dark", language: "en" });
const anotherConfigManager = ConfigurationManager.getInstance();
console.log(anotherConfigManager.getConfig()); // Output: { theme: "dark", language: "en" }
console.log(configManager === anotherConfigManager); // Output: true
Utilizing the Strategy Pattern
class ShippingStrategy {
calculateCost(item) {
throw new Error("Abstract method 'calculateCost' must be overridden");
}
}
class StandardShipping extends ShippingStrategy {
calculateCost(item) {
return item.weight * 2.5;
}
}
class ExpressShipping extends ShippingStrategy {
calculateCost(item) {
return item.weight * 5;
}
}
class ShoppingCart {
constructor(shippingStrategy) {
this.items = [];
this.shippingStrategy = shippingStrategy;
}
addItem(item) {
this.items.push(item);
}
calculateTotal() {
let total = 0;
for (const item of this.items) {
total += item.price;
}
total += this.shippingStrategy.calculateCost(this);
return total;
}
}
const standardShipping = new StandardShipping();
const expressShipping = new ExpressShipping();
const cart = new ShoppingCart(standardShipping);
cart.addItem({ price: 50, weight: 2 });
cart.addItem({ price: 30, weight: 1 });
console.log(`Total cost with standard shipping: $${cart.calculateTotal()}`);
// Output: Total cost with standard shipping: $137.5
cart.shippingStrategy = expressShipping;
console.log(`Total cost with express shipping: $${cart.calculateTotal()}`);
// Output: Total cost with express shipping: $147.5
Pitfalls to Avoid
- Overusing design patterns can lead to overly complex code.
- Selecting an inappropriate pattern for the problem at hand can hinder progress.
- Neglecting evolving requirements and rigidly adhering to patterns may result in suboptimal solutions.
8. Choosing the Right Design Pattern
Selecting the most suitable design pattern involves a thoughtful analysis of various factors.
Factors Influencing Pattern Selection
- Nature of the problem you're addressing.
- Project-specific requirements and constraints.
- Existing architectural design and infrastructure.
Adapting Patterns to Specific Scenarios
Design patterns are not rigid templates; they should be tailored to the specific context to achieve optimal outcomes.
9. Future Trends in JavaScript Design Patterns
As JavaScript continues to evolve, new design patterns may emerge to address emerging challenges and exploit new language features. Staying attuned to these trends is essential to staying ahead in the rapidly shifting landscape of web development.
10. Conclusion
The mastery of JavaScript design patterns equips developers with the tools to craft high-quality, maintainable, and scalable applications. By integrating these proven techniques into your development arsenal, you're poised to excel in the competitive realm of web development.
For more insights on boosting website traffic, visit The Insider's Views.
In conclusion, this Comprehensive Guide to JavaScript Design Patterns empowers you with a comprehensive understanding of each pattern, accompanied by detailed examples, setting you on a path to coding excellence and success.