- Published on
Demystifying the Publish-Subscribe Pattern in JavaScript
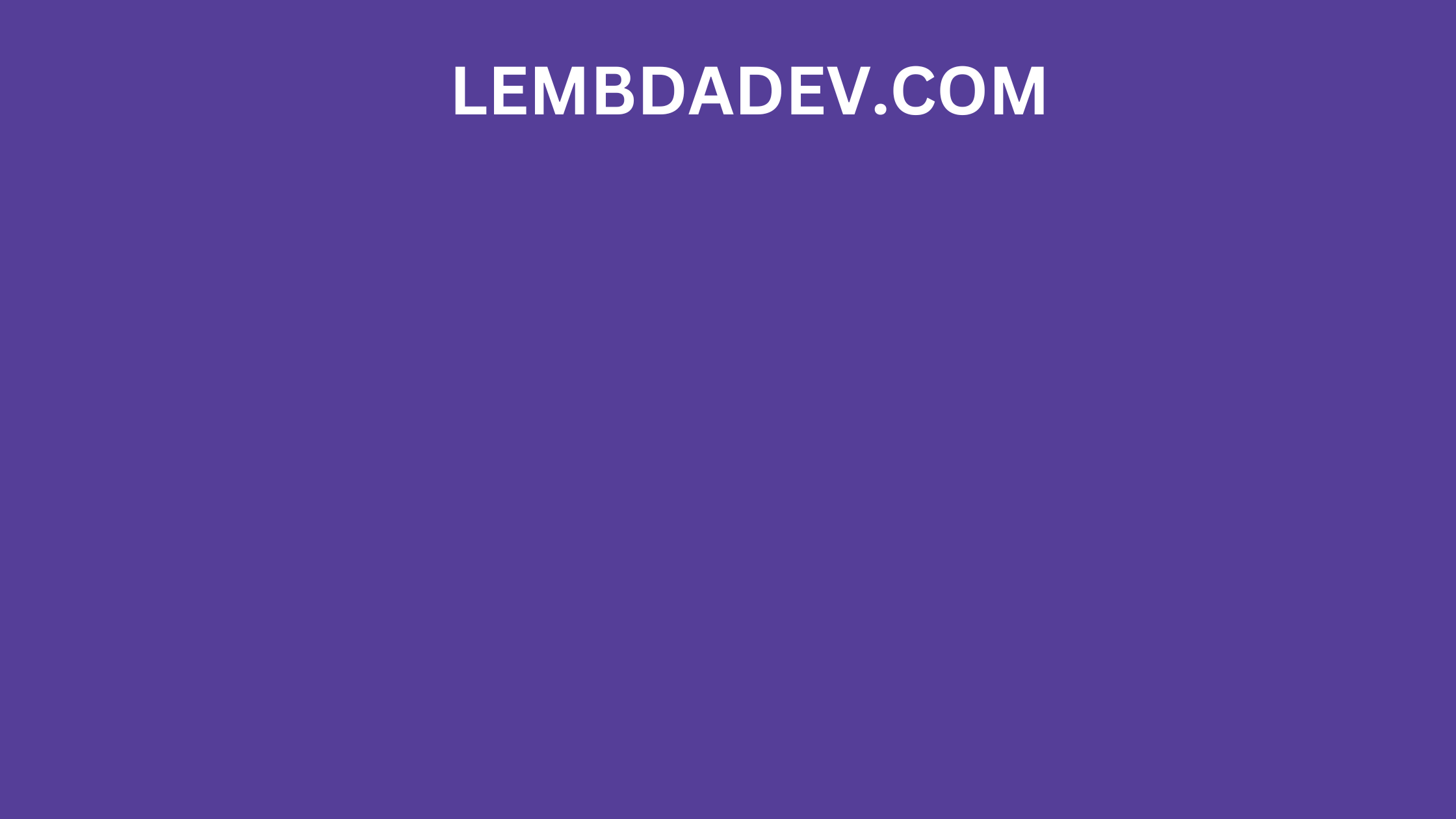
Demystifying the Publish-Subscribe Pattern in JavaScript
Exploring the Essence of the Publish/Subscribe Pattern
The Publish/Subscribe pattern, often referred to as Pub/Sub, is a behavioral design pattern that facilitates seamless communication between various components of an application. Unlike tightly coupled interactions, the Pub/Sub pattern fosters a decoupled approach, allowing components to communicate without having direct dependencies on each other.
Let's delve into the core concepts that underpin this pattern.
Key Components of the Pub/Sub Pattern
Publishers and Subscribers: A Dynamic Duo
At the heart of the Pub/Sub pattern are two pivotal actors: publishers and subscribers. Publishers are responsible for generating events, often encapsulating important data or updates. Subscribers, on the other hand, express interest in specific types of events and receive notifications when these events are published.
Message Broker: The Facilitator of Communication
The glue that holds the Pub/Sub pattern together is the message broker, sometimes referred to as the event bus. This intermediary entity receives events from publishers and then dispatches them to all interested subscribers. The message broker effectively eliminates direct coupling between publishers and subscribers, enabling a more modular and flexible architecture.
Unveiling the Advantages of the Pub/Sub Pattern
Loose Coupling for Enhanced Maintainability
The Pub/Sub pattern's hallmark is loose coupling. By reducing direct dependencies between components, changes made to one part of the application are less likely to impact others. This results in a more maintainable codebase and a smoother development experience.
Scalability and Extensibility: A Seamless Journey
As applications grow in complexity, the Pub/Sub pattern shines even brighter. New components can effortlessly join the ecosystem as subscribers to existing events or introduce fresh events, all without disrupting the existing codebase. This scalability and extensibility make the pattern a go-to choice for building robust applications.
Effective Communication: Preventing Information Silos
Pub/Sub promotes effective communication between disparate parts of an application. It helps prevent information silos by allowing components to share vital updates without needing intimate knowledge of each other's inner workings.
Bringing Pub/Sub to Life: Implementing the Pattern in JavaScript
Now that we've grasped the theoretical foundation, let's roll up our sleeves and explore how to implement the Pub/Sub pattern in JavaScript.
Step 1: Setting Up the Message Broker(Event Bus)
First, create a message broker. For our example, we'll use a simple custom implementation. You can extend this further by exploring libraries like PubSubJS
for advanced functionality.
class MessageBroker {
constructor() {
this.subscriptions = {};
}
subscribe(event, subscriber) {
if (!this.subscriptions[event]) {
this.subscriptions[event] = [];
}
this.subscriptions[event].push(subscriber);
}
publish(event, data) {
if (this.subscriptions[event]) {
this.subscriptions[event].forEach((subscriber) => subscriber(data));
}
}
}
const broker = new MessageBroker();
Step 2: Creating Publishers and Subscribers
Let's imagine a scenario where we have a weather application. We'll create a publisher that generates weather updates and subscribers that react to those updates.
// Publisher
function weatherPublisher(data) {
broker.publish("weatherUpdate", data);
}
// Subscribers
function temperatureSubscriber(data) {
console.log(`Temperature: ${data.temperature}°C`);
}
function humiditySubscriber(data) {
console.log(`Humidity: ${data.humidity}%`);
}
// Subscribing to events
broker.subscribe("weatherUpdate", temperatureSubscriber);
broker.subscribe("weatherUpdate", humiditySubscriber);
Step 3: Simulating Weather Updates
Now, let's simulate weather updates and see our subscribers in action.
// Simulating weather updates
setInterval(() => {
const weatherData = {
temperature: Math.random() * 30,
humidity: Math.random() * 100,
};
weatherPublisher(weatherData);
}, 5000);
In Conclusion
The Publish/Subscribe pattern is a potent tool in a JavaScript developer's toolbox, fostering modularity, scalability, and effective communication within applications. By embracing this pattern and leveraging its advantages, you can elevate your coding prowess and architect robust software solutions.