- Published on
How to type a Date object in TypeScript?
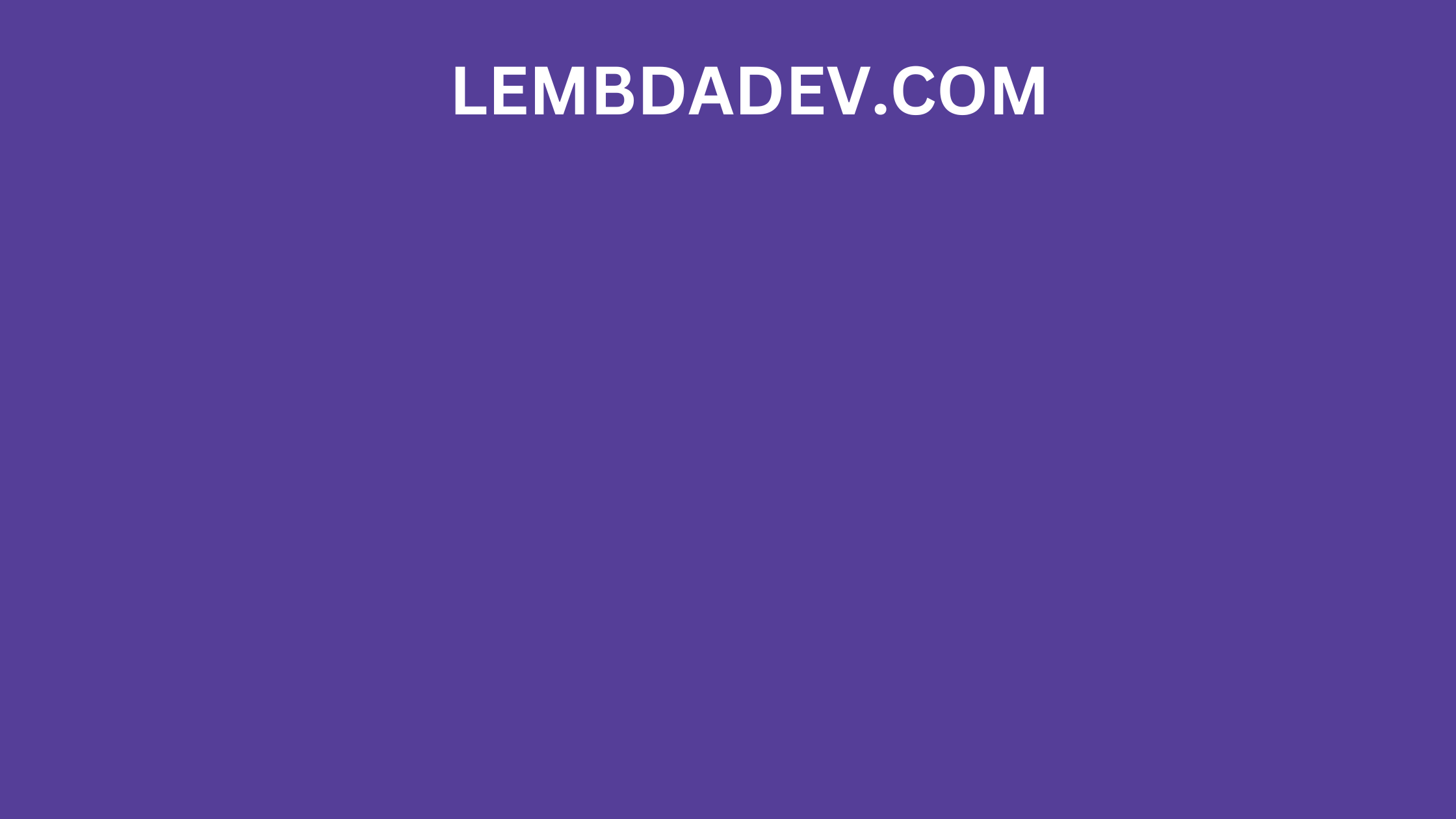
How to type a Date object in TypeScript?
How to type a Date object in TypeScript?
TypeScript is a typed superset of JavaScript that adds optional static type checking to the language. This means that TypeScript can help you catch errors in your code before you even run it. One of the benefits of using TypeScript is that it can help you type your Date objects. This can make your code more readable and maintainable.
What is a Date object?
A Date object represents a date and time in TypeScript. It has many properties and methods that can be used to manipulate dates and times.
How to type a Date object in TypeScript
There are two ways to type a Date object in TypeScript:
- Use the Date type: The Date type is a built-in TypeScript type that represents a Date object. To type a Date object using the Date type, simply declare a variable of type
Date
. For example:
const date: Date = new Date();
- Use a type interface: You can also use a type interface to type a Date object. This is useful if you want to define additional properties or methods for your Date object. For example, the following code defines a type interface called
MyDate
that extends the Date type:
interface MyDate extends Date {
// Additional properties or methods
}
To type a Date object using the MyDate
type interface, simply declare a variable of type MyDate
.
For example:-
const myDate: MyDate = new Date();
Using Date objects in TypeScript
Once you have typed a Date object, you can use it in your TypeScript code like any other object. However, it is important to remember that Date objects are immutable. This means that you cannot modify them directly. Instead, you need to use methods such as setDate()
, setMonth()
, and setFullYear()
to change the values of the Date object.
For example, the following code changes the date of the myDate
object to January 1, 2023:
myDate.setDate(1);
myDate.setMonth(0);
myDate.setFullYear(2023);
I hope this helps!
Related Artcles
- Type useState as Array or Object in React TypeScript
- Type the onFocus and onBlur events in React TypeScript
- Type onKeyDown, onKeyUp, onKeyPress events in React(TypeScript)
- Type the onClick event of an element in React(TypeScript)
- Type the onChange event of an element in React(TypeScript)
- Type the onSubmit event in React (TypeScript)
- Define types for process.env in TypeScript
- How to type an async Function in TypeScript
- How to Type the reduce() method in TypeScript
- How to Type the Spread method in TypeScript