- Published on
How to Type the onClick event of an element in React (TypeScript)?
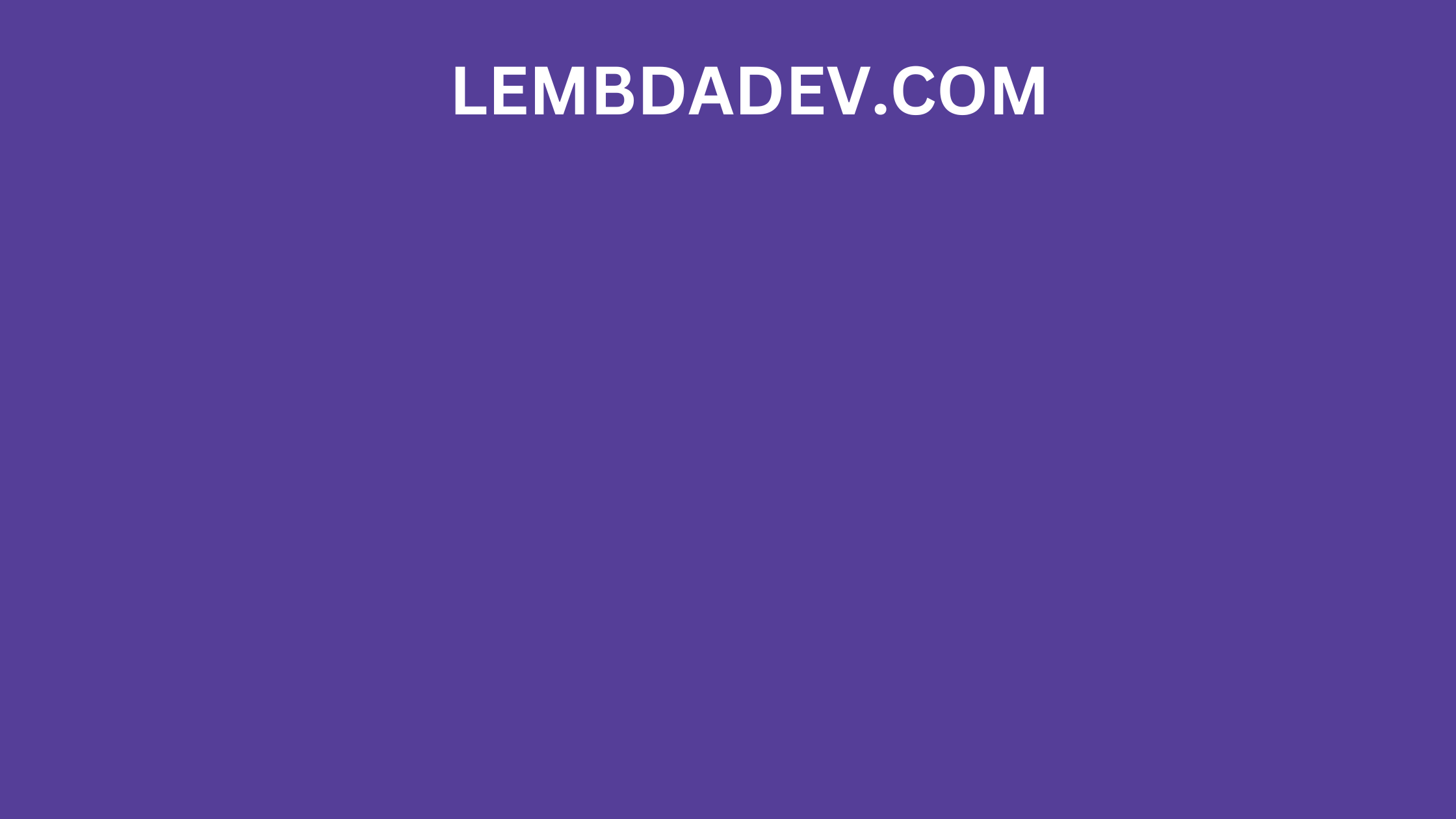
How to Type the onClick event of an element in React (TypeScript)?
How to Type the onClick event of an element in React (TypeScript)?
TypeScript is a typed superset of JavaScript that adds optional static type checking to the language. This means that TypeScript can help you catch errors in your code before you even run it. One of the benefits of using TypeScript is that it can help you type your event handlers. This can make your code more readable and maintainable.
What is the onClick event?
The onClick
event is a React event that is fired when an element is clicked. You can use the onClick
event to handle user interaction, such as opening a new window or submitting a form.
###How to type the onClick event in React (TypeScript)
To type the onClick
event in React (TypeScript), you need to specify the type of the event handler function. The type of the event handler function is always a function that takes an event object as an argument and returns void.
For example, the following code types the onClick
event handler function for a button element:
import React, { MouseEvent } from "react";
const Button: React.FC<{}> = () => {
const handleClick = (event: MouseEvent) => {
// Handle the click event
};
return <button onClick={handleClick}>Click me!</button>;
};
export default Button;
The handleClick()
function takes an event object as an argument and returns void. This means that the function can perform any operation that it needs to perform, such as opening a new window or submitting a form.
Using the onClick event in React (TypeScript)
Once you have typed the onClick
event handler function, you can use it in your React code like any other function. However, it is important to remember that the event handler function will be passed an event object as an argument. This means that you can use the event object to access information about the click
event, such as the target element and the coordinates of the click.
For example, the following code logs the target element of the click event to the console:
import React, { MouseEvent } from "react";
const Button: React.FC<{}> = () => {
const handleClick = (event: MouseEvent) => {
console.log(event.target);
};
return <button onClick={handleClick}>Click me!</button>;
};
export default Button;
When you click the button, the following output will be logged to the console:
<button onClick={handleClick}>Click me!</button>
I hope this is helpfull!
Related Artcles
- Type useState as Array or Object in React TypeScript
- Type the onFocus and onBlur events in React TypeScript
- Type onKeyDown, onKeyUp, onKeyPress events in React(TypeScript)
- Type the onChange event of an element in React(TypeScript)
- Type the onSubmit event in React (TypeScript)
- How to type a Date object in TypeScript
- Define types for process.env in TypeScript
- How to type an async Function in TypeScript
- How to Type the reduce() method in TypeScript
- How to Type the Spread method in TypeScript