- Published on
How to Type the onFocus and onBlur events in React TypeScript ?
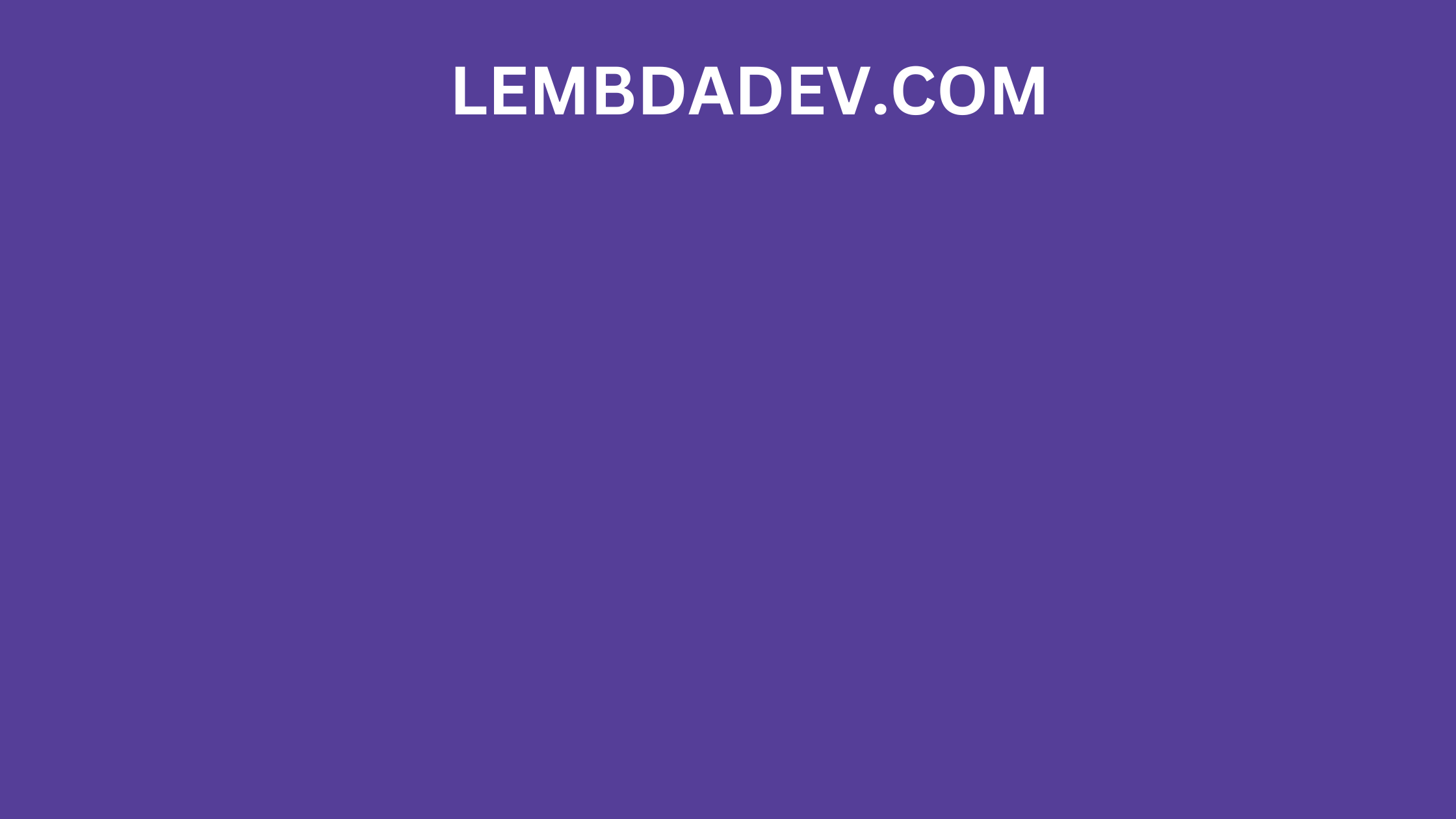
How to Type the onFocus and onBlur events in React TypeScript ?
How to Type the onFocus and onBlur events in React TypeScript?
TypeScript is a typed superset of JavaScript that adds optional static type checking to the language. This means that TypeScript can help you catch errors in your code before you even run it. One of the benefits of using TypeScript is that it can help you type your event handlers. This can make your code more readable and maintainable.
What are the onFocus and onBlur events?
The onFocus
and onBlur
events are React events that are fired when an element is focused or blurred, respectively. You can use these events to handle user interaction, such as showing or hiding a tooltip or updating the state of your component.
How to type the onFocus and onBlur events in React TypeScript
To type the onFocus
and onBlur
events in React TypeScript, you need to specify the type of the event handler function. The type of the event handler function is always a function that takes an event object as an argument and returns void.
For example, the following code types the onFocus
and onBlur
event handler functions for a text input element:
import React, { FocusEvent } from "react";
const Input: React.FC<{}> = () => {
const handleFocus = (event: FocusEvent<HTMLInputElement>) => {
// Handle the onFocus event
};
const handleBlur = (event: FocusEvent<HTMLInputElement>) => {
// Handle the onBlur event
};
return <input onFocus={handleFocus} onBlur={handleBlur} />;
};
export default Input;
The handleFocus()
and handleBlur()
functions take an event object as an argument and return void. This means that the functions can perform any operation that they need to perform, such as updating the state of the component or showing or hiding a tooltip.
Using the onFocus and onBlur events in React TypeScript
Once you have typed the onFocus
and onBlur
event handler functions, you can use them in your React code like any other function. However, it is important to remember that the event handler functions will be passed an event object as an argument. This means that you can use the event object to access information about the focus or blur event, such as the target element.
For example, the following code logs the target element of the focus
or blur
event to the console:
import React, { FocusEvent } from "react";
const Input: React.FC<{}> = () => {
const handleFocus = (event: FocusEvent<HTMLInputElement>) => {
console.log(event.target);
};
const handleBlur = (event: FocusEvent<HTMLInputElement>) => {
console.log(event.target);
};
return <input onFocus={handleFocus} onBlur={handleBlur} />;
};
export default Input;
When the user focuses or blurs the text input element, the following output will be logged to the console:
<input onFocus={handleFocus} onBlur={handleBlur} />
I hope this helps!
Related Artcles
- Type useState as Array or Object in React TypeScript
- Type onKeyDown, onKeyUp, onKeyPress events in React(TypeScript)
- Type the onClick event of an element in React(TypeScript)
- Type the onChange event of an element in React(TypeScript)
- Type the onSubmit event in React (TypeScript)
- How to type a Date object in TypeScript
- Define types for process.env in TypeScript
- How to type an async Function in TypeScript
- How to Type the reduce() method in TypeScript
- How to Type the Spread method in TypeScript