- Published on
How to sort a Set in JavaScript?
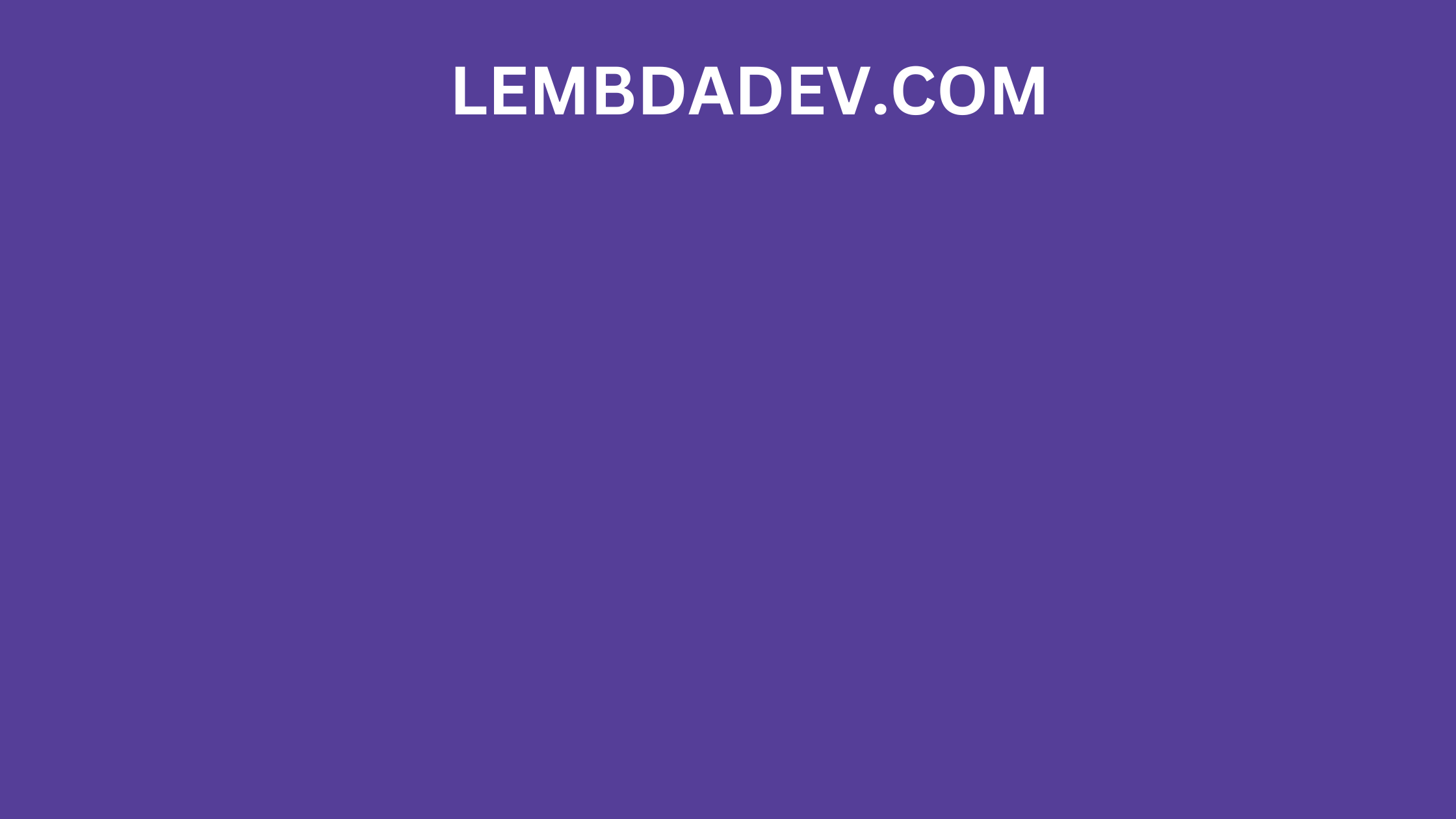
How to sort a Set in JavaScript?
How to sort a Set in JavaScript
Sets are a powerful data structure in JavaScript, but they are not ordered by default. This means that there is no built-in sort() method for Sets.
However, there are a few ways to sort a Set in JavaScript.
Array.from()
and Array.sort()
Method 1: Using The simplest way to sort a Set in JavaScript is to use the Array.from()
and Array.sort()
methods. The Array.from()
method converts a Set to an array, and the Array.sort()
method sorts the array in ascending order.
To sort a Set using this method, you would first use the Array.from()
method to convert the Set to an array. Then, you would use the Array.sort()
method to sort the array in ascending order. Finally, you would use the new Set() constructor to create a new Set from the sorted array.
Here is an example of how to sort a Set using the Array.from()
and Array.sort()
methods:
const set = new Set([5, 3, 1, 2, 4]);
// Convert the Set to an array.
const array = Array.from(set);
// Sort the array in ascending order.
`array.sort()`;
// Create a new Set from the sorted array.
const sortedSet = new Set(array);
// Log the sorted Set.
console.log(sortedSet); // Set { 1, 2, 3, 4, 5 }
Method 2: Using a custom sort function
Another way to sort a Set in JavaScript is to use a custom sort function. This method is more flexible than using the Array.from()
and Array.sort()
methods, but it is also more complex.
To sort a Set using a custom sort function, you would first create a function that compares two elements of the Set. This function should return a negative number if the first element is less than the second element, a positive number if the first element is greater than the second element, and zero if the two elements are equal.
Once you have created a custom sort function, you can use it to sort a Set using the Set.prototype.sort()
method. The Set.prototype.sort()
method takes a function as an argument, and it sorts the Set using the function to compare elements.
Here is an example of how to sort a Set using a custom sort function:
const set = new Set([5, 3, 1, 2, 4]);
// Create a custom sort function.
const sortFunction = (a, b) => a - b;
// Sort the Set using the custom sort function.
set.sort(sortFunction);
// Log the sorted Set.
console.log(set); // Set { 1, 2, 3, 4, 5 }
Which method should I use for sorting a Set?
The best method to use for sorting a Set in JavaScript depends on your specific needs. If you need a simple and straightforward way to sort a Set, then you should use the Array.from()
and Array.sort()
methods. If you need a more flexible way to sort a Set, then you should use a custom sort function.