- Published on
How to Split a String by Newline in JavaScript?
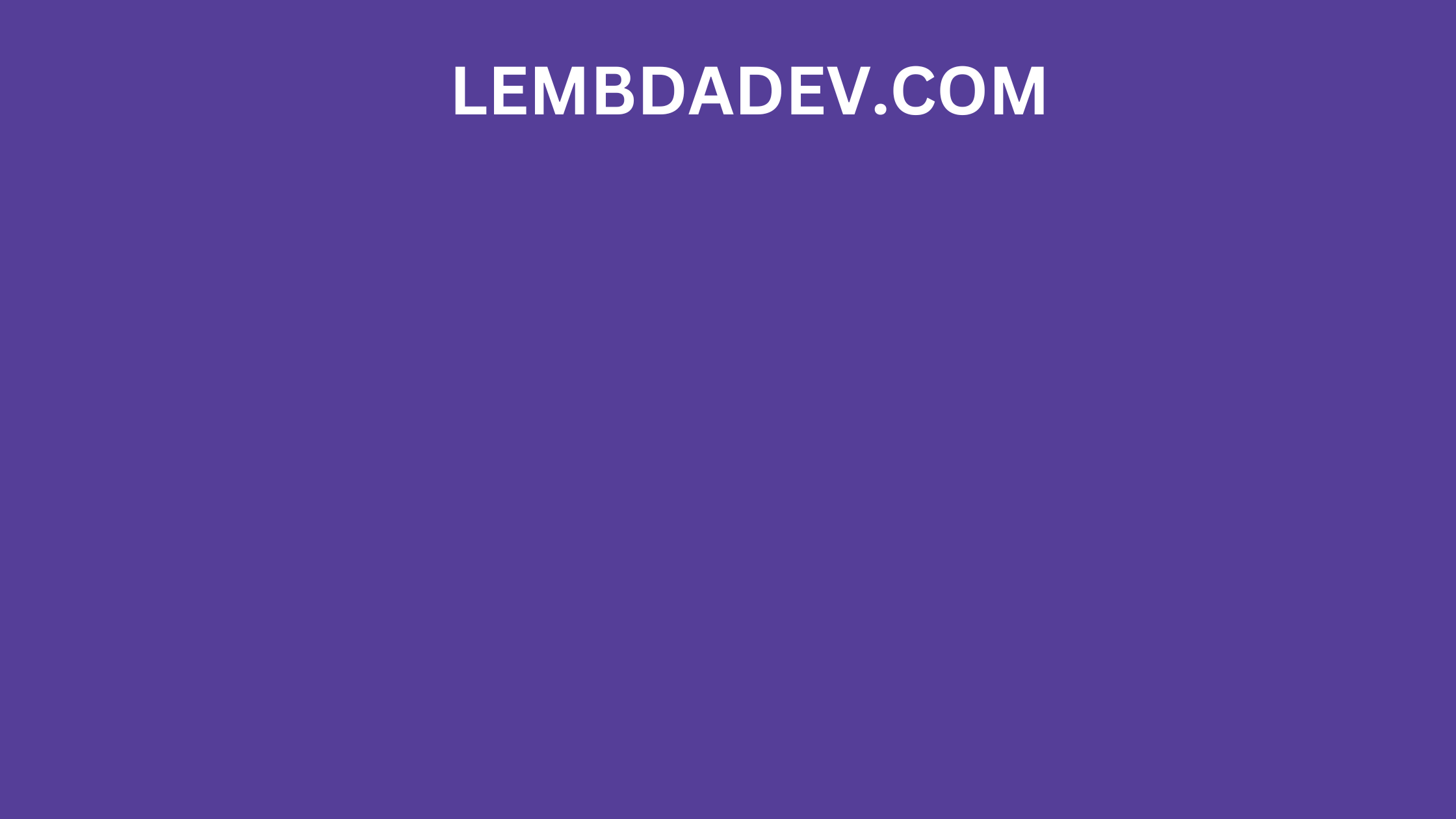
How to Split a String by Newline in JavaScript?
How to Split a String by Newline in JavaScript
JavaScript is a popular programming language that is used to create dynamic and interactive web pages. One common task in JavaScript is to split a string by newline. This can be useful for a variety of purposes, such as parsing user input, processing text data, or creating arrays of lines.
There are two main ways to split a string by newline in JavaScript:
string.split()
method
1. Use the The string.split()
method splits a string into an array of substrings, using a specified separator. To split a string by newline, you would pass the \n
character as the separator.
2. Use a regular expression
A regular expression is a sequence of characters that define a search pattern. You can use a regular expression to split a string by newline by matching the newline character.
Example:
// Split a string by newline using the string.split() method
const string = "This is a string\nwith multiple lines.";
const lines = string.split("\n");
console.log(lines); // ['This is a string', 'with multiple lines.']
// Split a string by newline using a regular expression
const regex = /\n/;
const lines2 = string.split(regex);
console.log(lines2); // ['This is a string', 'with multiple lines.']
Which method should I use?
The string.split()
method is generally considered to be the better method for splitting a string by newline. This is because it is more explicit and less likely to cause unexpected results. However, the regular expression method may be more useful in some cases, such as when you need to split a string by a specific pattern of newline characters.
Conclusion
Splitting a string by newline in JavaScript is a simple and straightforward process. By following the steps above, you can easily split any string into an array of lines.