- Published on
How to sort a Map in JavaScript?
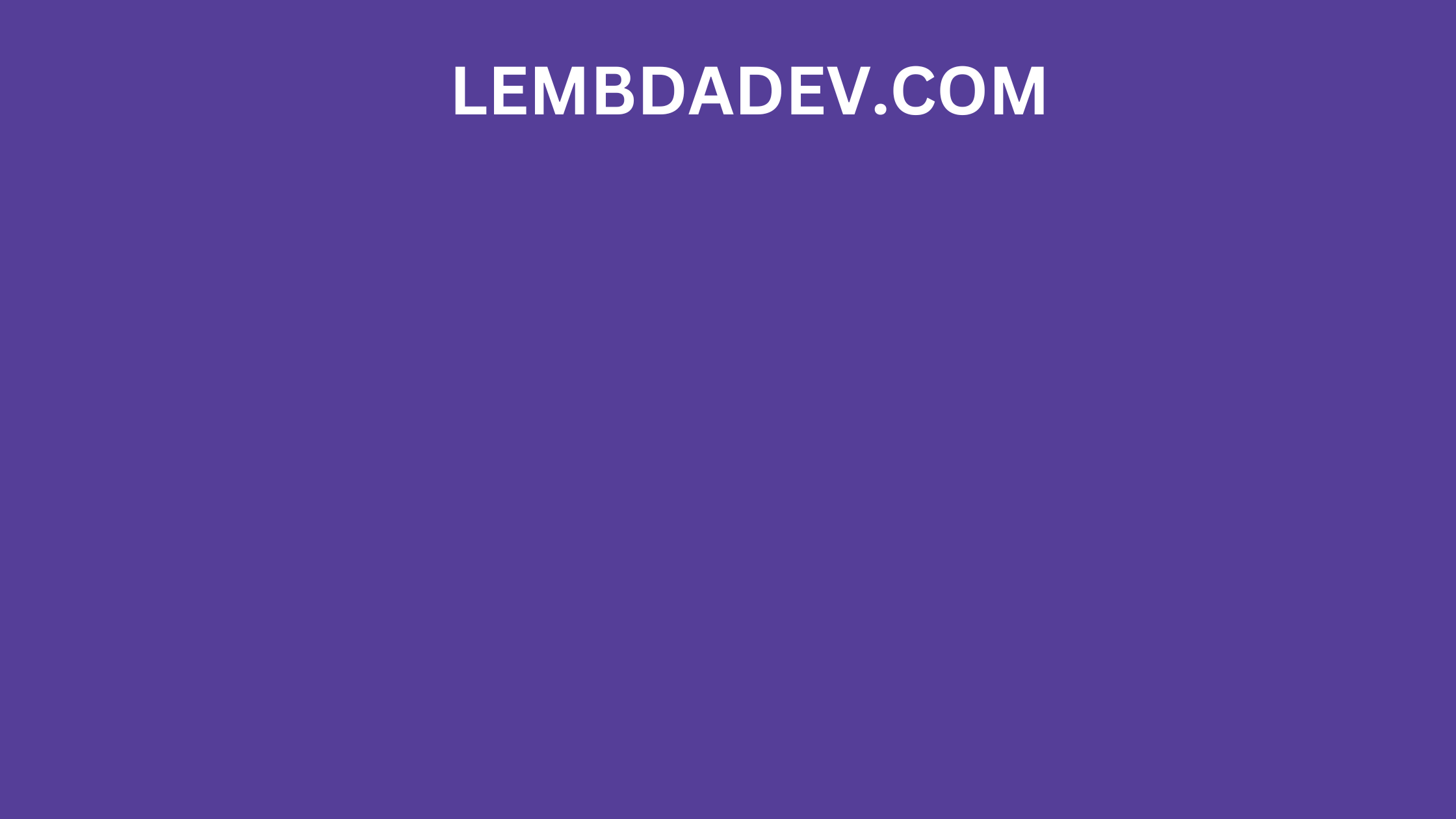
How to sort a Map in JavaScript?
How to Sort a Map in JavaScript
Maps are a powerful data structure in JavaScript, but they are not ordered by default. This means that there is no built-in sort() method for Maps.
However, there are a few ways to sort a Map in JavaScript.
Method 1: Using Array.from() and Array.sort()
The simplest way to sort a Map in JavaScript is to use the Array.from()
and Array.sort()
methods. The Array.from()
method converts a Map to an array, and the Array.sort()
method sorts the array in ascending order.
To sort a Map using this method, you would first use the Array.from()
method to convert the Map to an array. Then, you would use the Array.sort()
method to sort the array in ascending order. Finally, you would use the new Map() constructor to create a new Map from the sorted array.
Here is an example of how to sort a Map using the Array.from()
and Array.sort()
methods:
const map = new Map([
["name", "Alice"],
["age", 25],
["occupation", "Software Engineer"],
]);
// Convert the Map to an array.
const array = Array.from(map);
// Sort the array in ascending order.
array.sort((a, b) => a[0] > b[0]);
// Create a new Map from the sorted array.
const sortedMap = new Map(array);
// Log the sorted Map.
console.log(sortedMap); // Map { age: 25, name: 'Alice', occupation: 'Software Engineer' }
Method 2: Using a custom sort function
Another way to sort a Map in JavaScript is to use a custom sort function. This method is more flexible than using the Array.from()
and Array.sort()
methods, but it is also more complex.
To sort a Map using a custom sort function, you would first create a function that compares two elements of the Map. This function should return a negative number if the first element is less than the second element, a positive number if the first element is greater than the second element, and zero if the two elements are equal.
Once you have created a custom sort function, you can use it to sort a Map using the Map.prototype.sort()
method. The Map.prototype.sort()
method takes a function as an argument, and it sorts the Map using the function to compare elements.
Here is an example of how to sort a Map using a custom sort function:
const map = new Map([
["name", "Alice"],
["age", 25],
["occupation", "Software Engineer"],
]);
// Create a custom sort function.
const sortFunction = (a, b) => a[0].localeCompare(b[0]);
// Sort the Map using the custom sort function.
map.sort(sortFunction);
// Log the sorted Map.
console.log(map); // Map { age: 25, name: 'Alice', occupation: 'Software Engineer' }
Which method should I use for sorting a Map in JavaScript?
The best method to use for sorting a Map in JavaScript depends on your specific needs. If you need a simple and straightforward way to sort a Map, then you should use the Array.from()
and Array.sort()
methods. If you need a more flexible way to sort a Map, then you should use a custom sort function.