- Published on
How to skip over an Element or an Index in .map() in JS
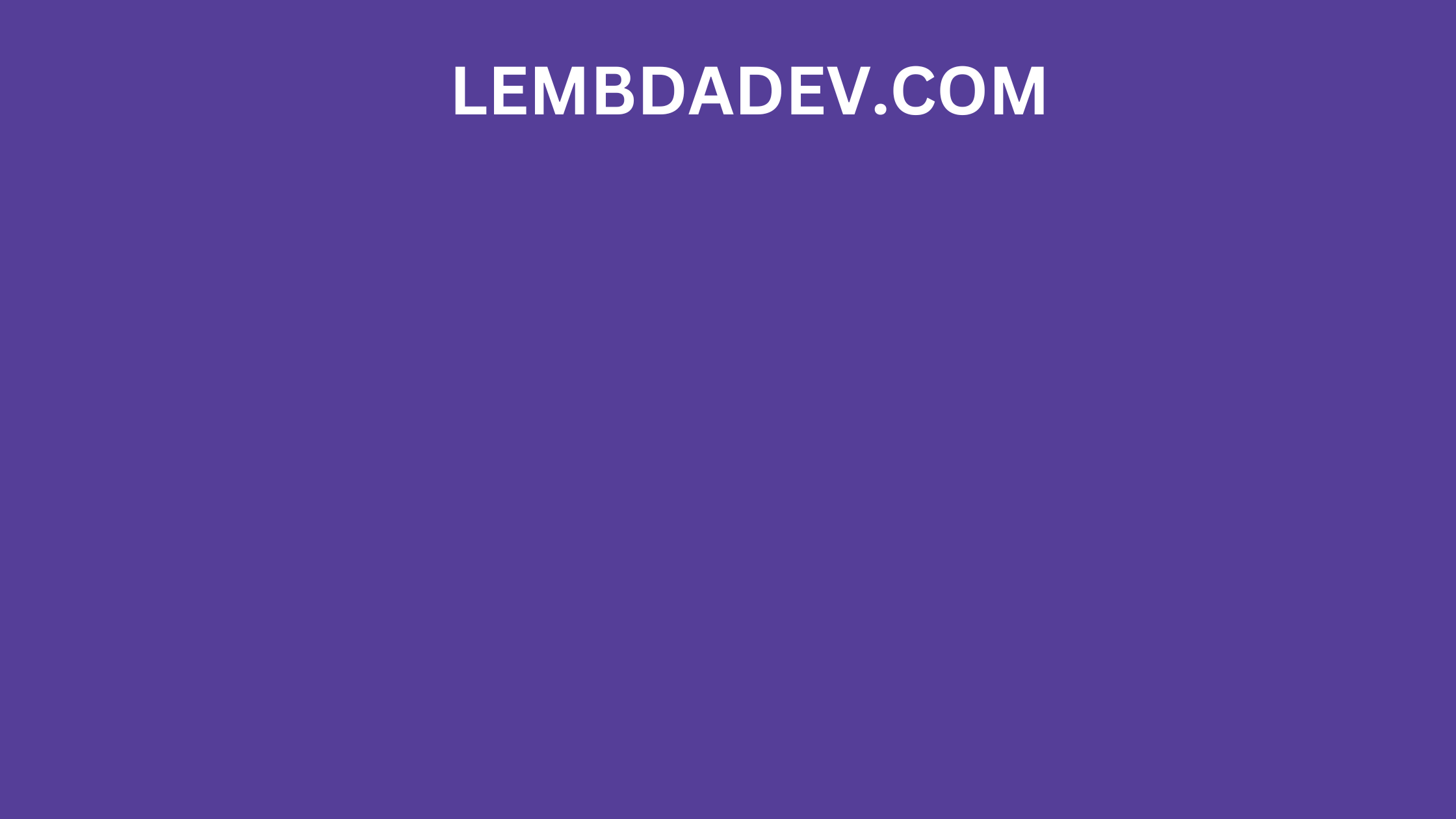
How to skip over an Element or an Index in .map() in JS
How to skip over an Element or an Index in .map() in JS
There is no direct way to skip over an element or index in the .map()
method in JavaScript. However, there are a few workarounds that you can use:
if
statement
1. Use the You can use the if
statement to check if the element or index that you want to skip matches a certain condition. If it does, you can return undefined
. Otherwise, you can return the element or index.
Here is an example:
const array = [1, 2, 3, 4, 5];
// Skip over the element at index 2.
const mappedArray = array.map((element, index) => {
if (index === 2) {
return undefined;
} else {
return element;
}
});
console.log(mappedArray); // [1, 2, undefined, 4, 5]
filter()
method
2. Use the You can use the filter()
method to filter out the element or index that you want to skip. Then, you can use the .map()
method to map over the remaining elements in the array.
Here is an example:
const array = [1, 2, 3, 4, 5];
// Skip over the element at index 2.
const mappedArray = array
.filter((element, index) => index !== 2)
.map((element) => element);
console.log(mappedArray); // [1, 2, 4, 5]
reduce()
method
3. Use the You can use the reduce()
method to create a new array from the original array, skipping over the element or index that you want to skip.
Here is an example:
const array = [1, 2, 3, 4, 5];
// Skip over the element at index 2.
const mappedArray = array.reduce((accumulator, element, index) => {
if (index !== 2) {
accumulator.push(element);
}
return accumulator;
}, []);
console.log(mappedArray); // [1, 2, 4, 5]
Which method to use
The best method to use to skip over an element or index in the .map()
method depends on your specific needs. If you need to skip over a specific element or index, you can use the if
statement. If you need to skip over multiple elements or indices, you can use the filter()
or reduce()
method.
Here is a table that summarizes the three methods:
Method | Description |
---|---|
if statement | Checks if the element or index matches a certain condition. If it does, returns undefined . Otherwise, returns the element or index. |
filter() method | Filters out the element or index that you want to skip. Then, maps over the remaining elements in the array. |
reduce() method | Creates a new array from the original array, skipping over the element or index that you want to skip. |
I hope this helps!