- Published on
How to Escape Quotes in a String using JavaScript?
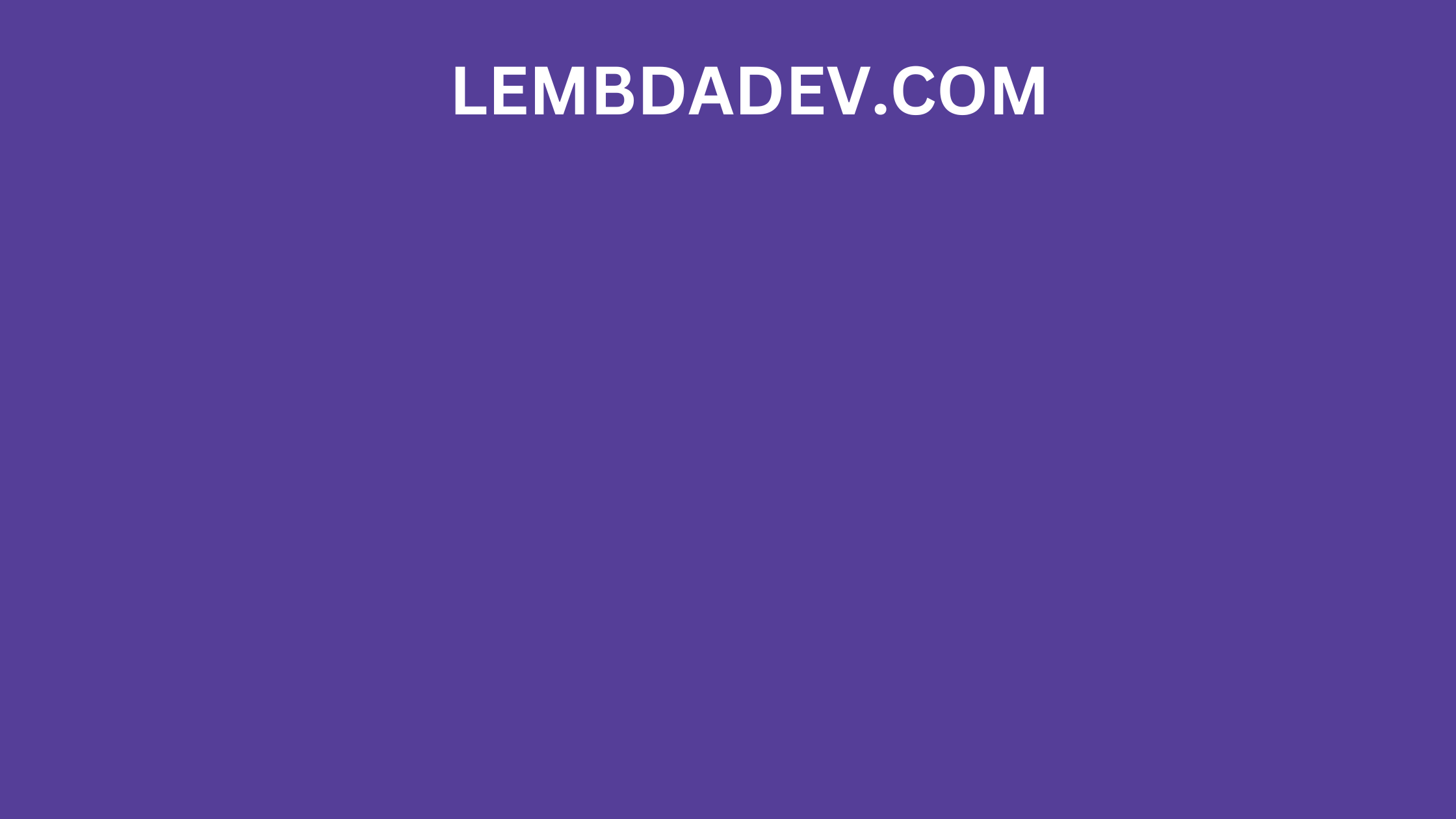
How to Escape Quotes in a String using JavaScript?
How to Escape Quotes in a String using JavaScript
Escape characters
Escape characters are used to tell JavaScript how to interpret certain characters in a string. For example, the backslash (\
) character is used to escape quotes, which tells JavaScript to treat the following quote as a literal character instead of the end of the string.
Escape quotes in a string
To escape quotes in a string using JavaScript, simply place a backslash (\
) character in front of the quote. For example:
const stringWithQuotes = 'This is a string with "quotes".';
// Escape the quotes in the string.
const escapedString = stringWithQuotes.replace(/"/g, '\\"');
// Log the escaped string.
console.log(escapedString); // "This is a string with \"quotes\"."
Escape quotes in a template literal
Template literals are a newer way to create strings in JavaScript. They allow you to embed expressions in strings, and they also make it easy to escape quotes.
To escape quotes in a template literal, simply place a dollar sign ($
) character in front of the quote. For example:
// Create a template literal.
const templateLiteral = `This is a string with ${'"quotes"}`;
// Log the template literal.
console.log(templateLiteral); // "This is a string with \"quotes\""
When to escape quotes
You should escape quotes in a string whenever you need to include a quote as a literal character in the string. For example, if you are creating a string that represents a JSON object, you will need to escape the quotes in the object keys and values.
You should also escape quotes if you are creating a string that will be injected into HTML code. This is because HTML interprets quotes as the beginning and end of attribute values.
Conclusion
Escaping quotes in a string is a simple way to ensure that your strings are interpreted correctly by JavaScript. You can escape quotes using the backslash (\
) character or by using a template literal.