- Published on
How to import JavaScript file into a TypeScript file?
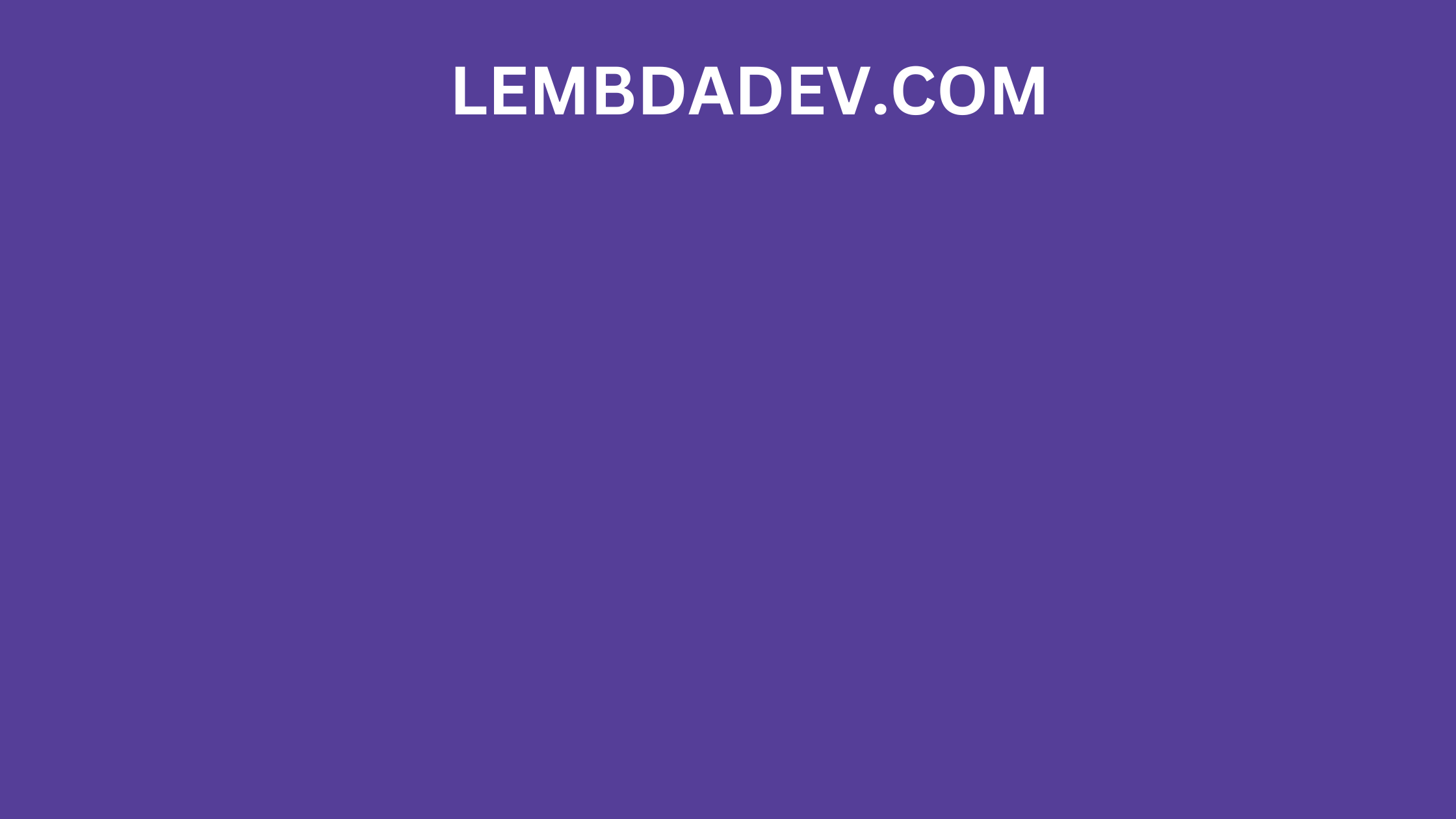
How to import JavaScript file into a TypeScript file?
How to import JavaScript file into a TypeScript file?
To import a JavaScript file into a TypeScript file, you can use the following steps:
tsconfig.json
file. Add the following line to the compilerOptions
section:
1. Enable JavaScript imports in your
"allowJs": true,
2. Import the JavaScript file using the following syntax:
import * as <module-name> from './<path-to-file>';
For example, to import the JavaScript file employee.js
, you would use the following code:
import * as Employee from "./employee.js";
Employee
class:
3. Use the imported module in your TypeScript code. For example, you could create a new instance of the const employee: Employee = new Employee();
If you are importing a specific export from a JavaScript file, you can use the following syntax:
import { <export-name> } from './<path-to-file>';
For example, to import the name
property from the Employee
class, you would use the following code:
import { name } from "./employee.js";
Once you have imported a JavaScript file into a TypeScript file, you can use it in your code just like any other TypeScript module.
Example of a complete TypeScript file that imports a JavaScript file
// Import the `Employee` class from the `employee.js` file.
import { Employee } from "./employee.js";
// Create a new instance of the `Employee` class.
const employee: Employee = new Employee();
// Log the employee's name to the console.
console.log(employee.name);
This code will compile and run without any errors.
Note: If you are using TypeScript to compile your code to JavaScript, you will need to make sure that the JavaScript file that you are importing is also compiled to JavaScript. You can do this by using the npm run build
command or by using a tool such as Webpack.