- Published on
How to Create a Date without Timezone in JavaScript?
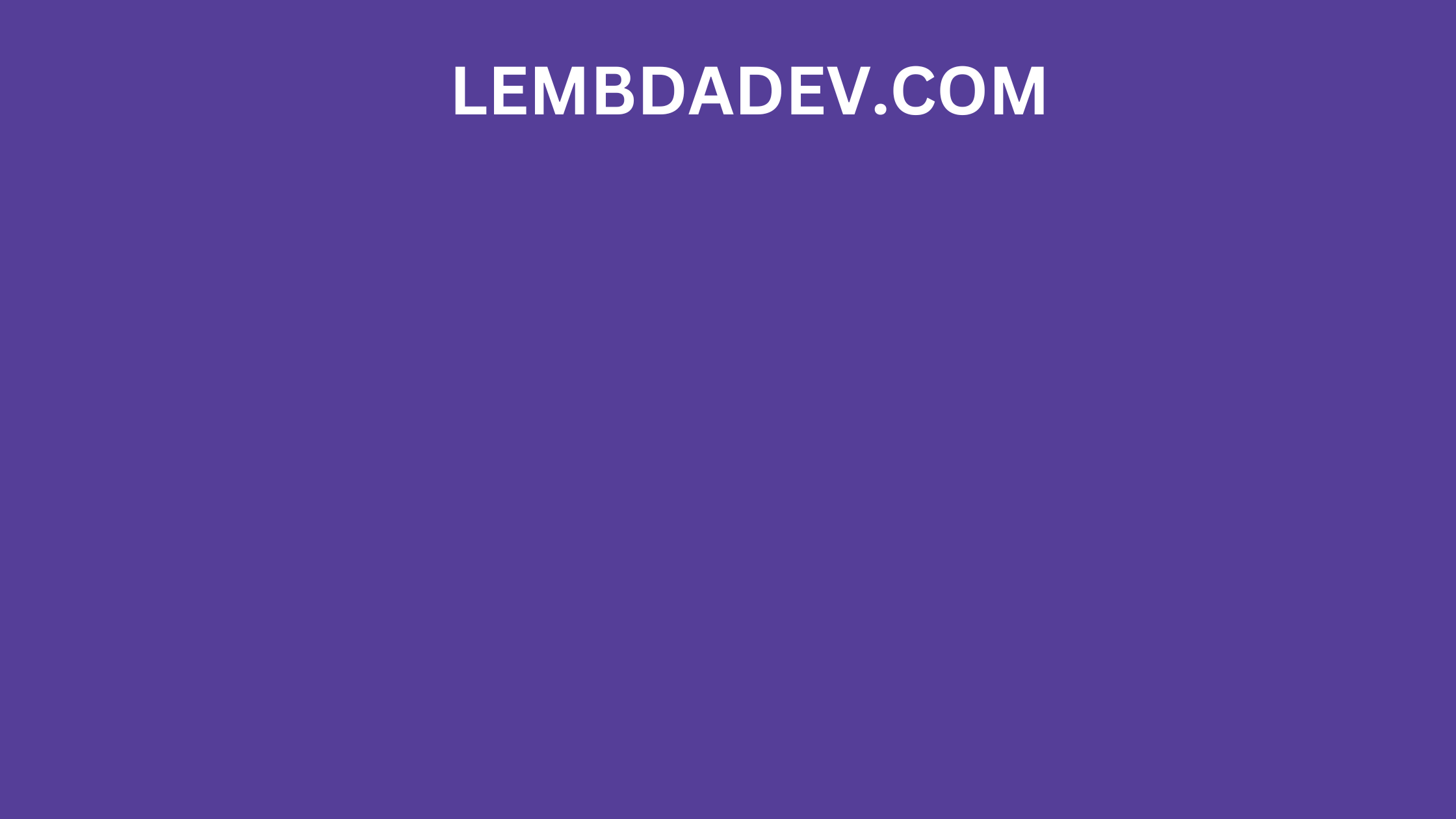
How to Create a Date without Timezone in JavaScript?
How to Create a Date without Timezone in JavaScript
JavaScript Date object
The JavaScript Date object represents a date and time. It has a number of methods and properties that can be used to manipulate and format dates.
However, the Date object always has a timezone associated with it. This is because JavaScript dates are based on the Unix epoch, which is the number of milliseconds that have elapsed since midnight Coordinated Universal Time (UTC) on January 1, 1970.
This can be a problem if you need to work with dates without a timezone, such as when storing dates in a database or sending them over a network.
Create a Date object without timezone
There are two ways to create a Date object without timezone in JavaScript:
Use the toISOString() method. The toISOString() method returns a string representation of the Date object in ISO 8601 format. This format is timezone-agnostic, so it can be used to represent dates without a timezone.
Manually set the hours and minutes. The Date object has a number of methods that can be used to set the hours, minutes, seconds, and milliseconds of the date. To create a Date object without timezone, you can manually set the hours and minutes to 0.
Example to Create a Date without Timezone in JavaScript
// Create a Date object with a timezone.
const dateWithTimezone = new Date();
// Get the ISO representation of the date.
const isoDateString = dateWithTimezone.toISOString();
// Remove the Z character from the ISO string to remove the timezone.
const isoStringWithoutTimezone = isoDateString.substring(
0,
isoDateString.length - 1
);
// Create a new Date object from the ISO string without timezone.
const dateWithoutTimezone = new Date(isoStringWithoutTimezone);
// Log the date objects.
console.log(dateWithTimezone); // 2023-09-28T18:07:41.000Z
console.log(dateWithoutTimezone); // 2023-09-28T00:00:00.000
Use a library to Create a Date without Timezone in JavaScript
There are also a number of JavaScript libraries that can be used to create and manipulate dates without timezones. One popular library is Moment.js.
To create a Date object without timezone using Moment.js, you can use the following code:
// Import the Moment.js library.
import moment from "moment";
// Create a Moment.js object without timezone.
const dateWithoutTimezone = moment().utcOffset(0);
// Get the Date object from the Moment.js object.
const dateWithoutTimezoneJS = dateWithoutTimezone.toDate();
Conclusion
There are two ways to create a Date object without timezone in JavaScript:
- Use the
toISOString()
method. - Manually set the hours and minutes to 0.
You can also use a JavaScript library such as Moment.js
to create and manipulate dates without timezones.
Which method you choose will depend on your specific needs. If you need to create a Date object without timezone from an ISO string, you can use the toISOString()
method. If you need to create a Date object without timezone from a Unix timestamp, you can manually set the hours and minutes to 0.
If you need to work with dates without timezones on a regular basis, you may want to consider using a JavaScript library such as Moment.js
. Moment.js
provides a number of features for working with dates and times, including the ability to create and manipulate dates without timezones.