- Published on
How to Create a Video Element using JavaScript?
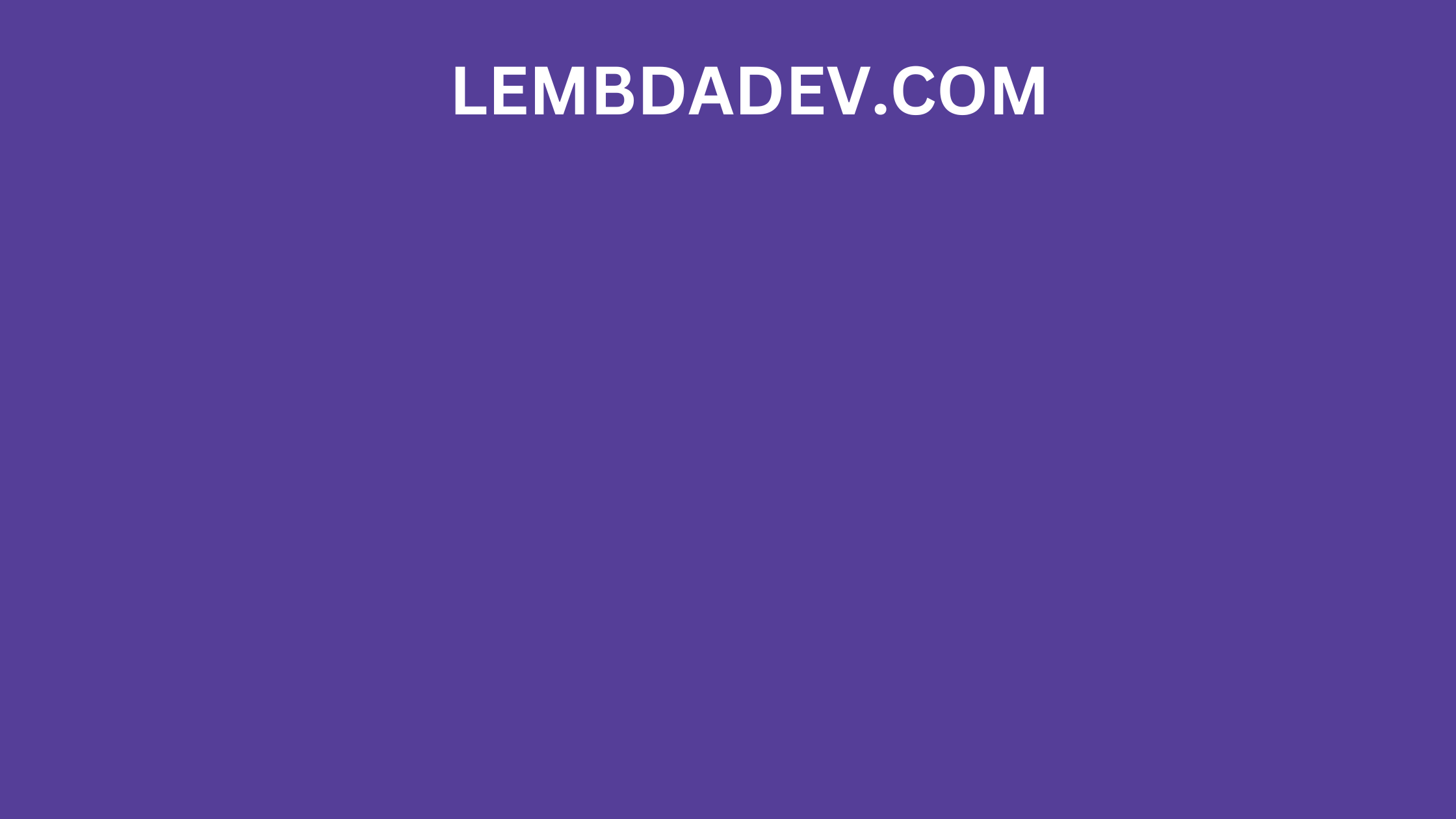
How to Create a Video Element using JavaScript?
How to Create a Video Element using JavaScript
JavaScript is a powerful language that can be used to manipulate web pages in a variety of ways. One common task is to create and manage video elements. This can be useful for a variety of purposes, such as embedding videos in web pages or creating video players.
To create a video element using JavaScript, you can use the document.createElement()
method. This method creates a new HTML element, based on the specified tag name. To create a video element, you would pass the video
tag name to the document.createElement()
method.
Common Video attributes
Once you have created the video element, you need to set its attributes. Some common attributes that you may want to set include:
- src: The URL of the video file.
- autoplay: Whether or not the video should start playing automatically when it is loaded.
- controls: Whether or not the video should display playback controls.
- width: The width of the video.
- height: The height of the video.
You can also set other attributes, such as the poster
attribute to specify a poster image for the video, or the loop
attribute to specify whether or not the video should loop when it reaches the end.
Once you have set the video element's attributes, you can append it to the DOM using the appendChild()
method. This will add the video element to the web page.
Example:
// Create a video element
const video = document.createElement("video");
// Set the video's attributes
video.src = "https://example.com/video.mp4";
video.autoplay = true;
video.controls = true;
video.width = 320;
video.height = 240;
// Append the video element to the DOM
document.body.appendChild(video);
This code will create a video element and append it to the body of the web page. The video will start playing automatically and will have playback controls.
Controlling the video element
Once you have created a video element, you can use JavaScript to control it. For example, you can use the play()
and pause()
methods to control playback, the currentTime
property to get the current playback position, and the duration
property to get the total duration of the video.
You can also use event listeners to listen for events on the video element, such as the play
event, the pause
event, and the ended
event. This allows you to perform actions when these events occur.
Example:
// Add an event listener for the play event
video.addEventListener("play", function () {
// Do something when the video starts playing
});
// Add an event listener for the pause event
video.addEventListener("pause", function () {
// Do something when the video is paused
});
// Add an event listener for the ended event
video.addEventListener("ended", function () {
// Do something when the video reaches the end
});
Creating a custom video player
You can use JavaScript to create your own custom video player. This can be useful if you need more control over the video player than the default controls provide.
To create a custom video player, you would first need to create a container element to hold the video element. You could then use CSS to style the container element and position it on the web page.
Next, you would need to add the video element to the container element. You could then use JavaScript to add custom playback controls to the container element.
For example, you could add a button to play and pause the video, a button to rewind the video, and a button to fast forward the video. You could also use JavaScript to display the current playback position and the total duration of the video.
Once you have added the custom playback controls to the container element, you can append the container element to the DOM. This will add the custom video player to the web page.
Conclusion
Creating a video element using JavaScript is a simple and straightforward process. By following the steps above, you can easily create and manage video elements on your web pages. You can also use JavaScript to create your own custom video players.