- Published on
Var, Let, and Const – What's the Difference?
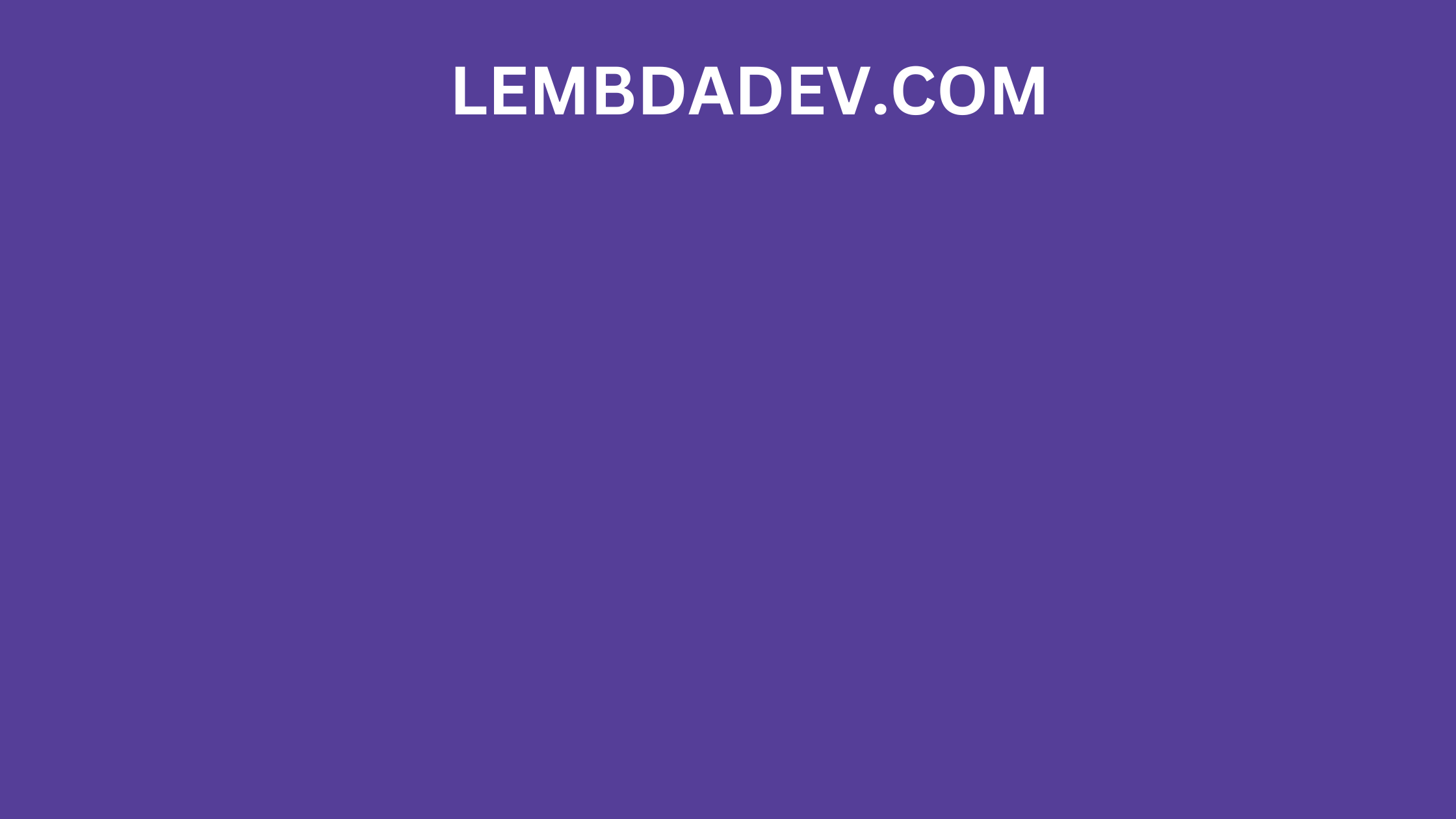
Var, Let, and Const – What's the Difference?
Variables in Javascript
With the introduction of ES2015 (ES6) a lot of shiny new features came out. One of the features that came with ES6 is how we declare variables in javascript. Earlier we have Var for variable declaration, with ES6 we have two new way to declare variable using let and const. Javascript provides us with multiple ways to declare a variable, but which one we should use, it depends on how we want to use and where we want to use it.
var
In Javascript?
What is Before the introduction of ES6, var
is used for variable declaration. var
variable has the function scope, But global scope when it is declared outside the function and When we declare a var
variable, it gets hoisted to the top of the scope and get assigned with the value of undefined
Example of var variable with function scope:
function fn() {
var fnscope = "function scope";
console.log(fnscope);
// function scope (Print 'function scope' as this is declared inside fn function)
}
fn(); //output => "my name"
console.log(fnscope);
//output=> Uncaught ReferenceError: fnscope is not defined
//Explaination: fnscope variable not declared inside of fn function so it will be undefined outside fn function scope
Example of var variable with Global scope:
var city = "Bengalore";
function fn() {
console.log(city);
//output => Bengalore
//city is declared outside the function body, and that's why having global scope.
}
console.log(city);
//output => Bengalore
Example of Hoisting of var Variable:
Hosting is javascript concept where variables and function declarations are moved to the top of their scope before code execution. This means that if we do this: Want to know more about Hoisting read it here.
console.log(name); // undefined
var name = "Neeraj";
console.log(name); // Neeraj
// After hoisting it will be look like this -
var name; //=> variable gets hoisted to the top of the scope and initialized with undefined
console.log(name); // undefined
name = "Neeraj"; //initialization of the value happens where the variable was declare
console.log(name); // Neeraj
Redeclaration of var Variable:
var
variable can be re-declared and updated. re-declaration allows declaring more than one variable with the same name, because of this, if we declare a new variable by mistake, it will override the original variable value.
Lets take one example to understand this concept -
var myName = "Sachin";
var age = 20;
console.log(myName);
function fn() {
var myName = "Dinesh";
console.log(myName); //Dinesh
// Local variable override global variable
}
fn();
if (age == 20) {
var myName = "Virat";
// it will override the original value
}
console.log(myName); //Virat
let
In Javascript?
What is let
is new way to declare variable in javascript(ES6) and an improvement on var
. It can be updated within its scope but does not allow re-declaration of variables and if we redeclare any variable in code it will through SyntaxError: redeclaration of let
const
In Javascript?
What is const
behave similar to let
, only difference is you can’t update it’s value after declaration.
const message = "Hello, Good Morning";
const message = "Hi, Good Morning";
// error: Identifier 'message' has already been declared
Scope of let and Const variable
let
and const
variable have block scope, which means these variable will be accessible only inside the block in which it declared, if we try of access outside of the scope it will through Reference Error.
Hoisting of let and const
let
and const
declarations are hoisted to the top but unlike var
which is initialized as undefined
,let
and const
keywords are not initialized. So if you try to use a let
and const
variables before declaration, you'll get a Reference Error
.
console.log(name); //output => ReferenceError
let name = "Virat";
Comparison between Var, Let and Const
Property | var | let | const |
---|---|---|---|
Scope | function or global scope. | block scope. | block scope. |
Hoisting | It gets hoisted to the top of its scope and initialized undefined. | It also got hoisted to the top of its scope but didn't initialize. | It also got hoisted to the top of its scope but didn't initialize. |
Updation | It can be updated or re-declared. | It can only be updated and can't be re-declared. | It can't be updated or re-declared. |