- Published on
JavaScript Hoisting and Temporal Dead Zone(TDZ)
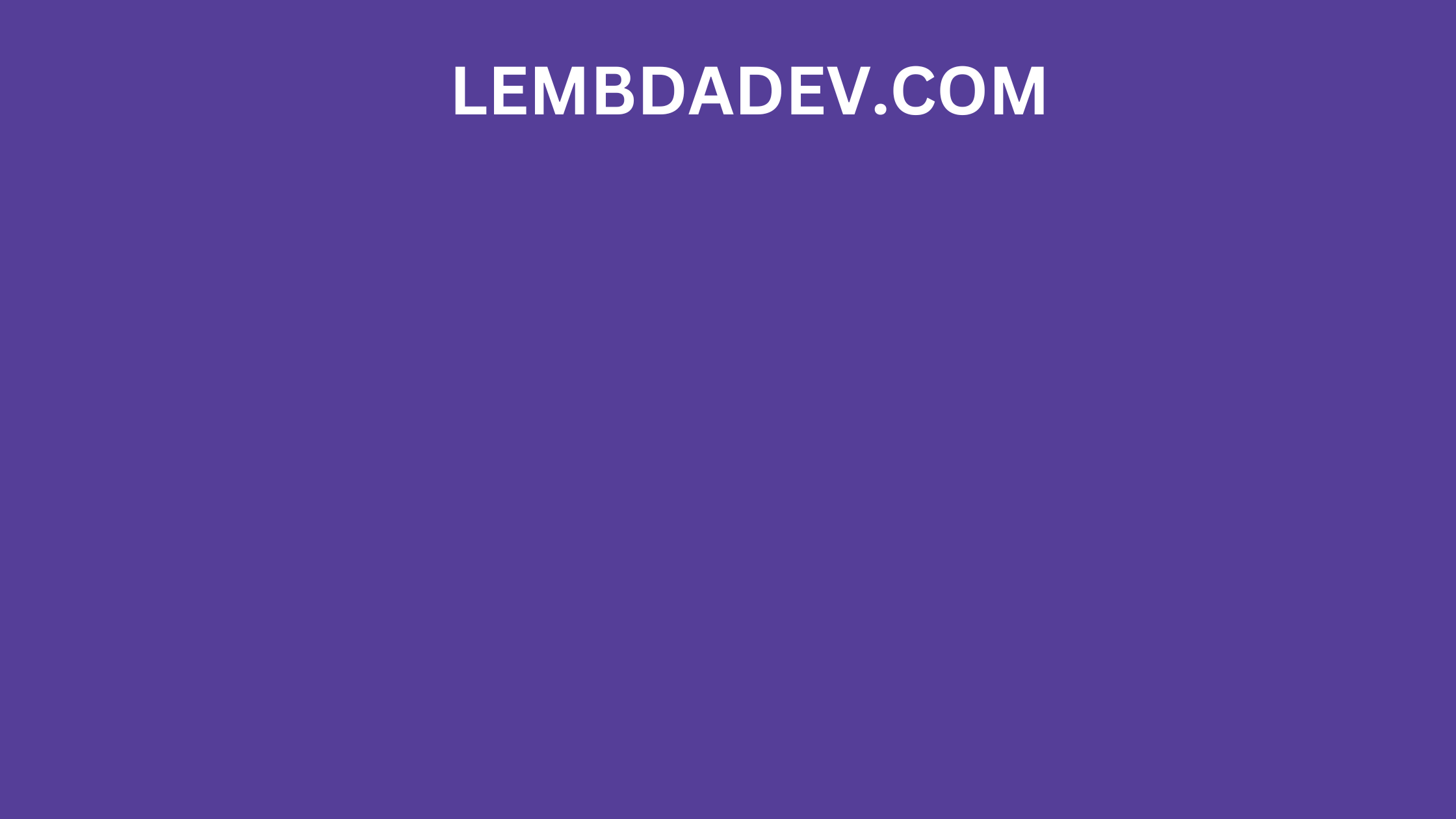
JavaScript Hoisting and Temporal Dead Zone(TDZ)
Hoisting & Temporal Dead Zone in Javascript
Temporal Dead Zone and Hoisting are two essential terms in JavaScript to understand how variables got declared and initialized. Here we will going to discuss it in details -
What is Hoisting in Javascript?
JavaScript Hoisting refers to the process whereby the interpreter appears to move the declaration of functions, variables or classes to the top of their scope, prior to execution of the code.
In other words Hoisting is a JavaScript mechanism where variables and function declarations are moved to the top of their scope before code execution, regardless of whether their scope is global or local.
Hoisting mechanism only moves the declaration not the initialization.
What is Variable Hoisting
The scope of a variable declared with the keyword var is its current execution context. This is either the enclosing function or for variables declared outside any function, global.
// Below two codes will work in same way because of hoisting
console.log(hoist); // Output: undefined
var hoist = 'The variable has been hoisted.';
console.log(hoist); //The variable has been hoisted
--------------------------------------------------------------------
var hoist;
console.log(hoist); // Output: undefined
hoist = 'The variable has been hoisted.';
console.log(hoist); //The variable has been hoisted
What is Function Hoisting?
JavaScript functions are classified as the following:
Function declarations
Function declarations are hoisted completely to the top. So we can invoke a function before declaring it.
hoisted(); // Output: "This function has been hoisted." function hoisted() { console.log("This function has been hoisted."); }
Function expressions
Function expressions are not hoisted, and the interpreter throws a
TypeError
since it seesexpression
as a variable and not a function.expression(); //Output: "TypeError: expression is not a function var expression = function () { console.log("Will this work?"); };
Things To Remember:
- Variable assignment takes precedence over function declaration
- Function declarations take precedence over variable declarations
What is Temporal Dead Zone(TDZ) in Javascript?
In Javascript we can declare variable using let
, const
and var
and the difference among these is let
and const
have block scope while var
have Global/function scope.
Second difference is var is hoisted and initialize with undefined while are also hoisted but there is a period between entering scope and being declared where they cannot be accessed. This period is the temporal dead zone (TDZ).
Temporal Dead Zone relates to time, not the space above the declaration of let
or cons
.
console.log(aVar); // undefined
console.log(aLet); // Causes ReferenceError: Cannot access 'aLet' before initialization in TDZ
var aVar = 1;
let aLet = 2;