- Published on
How to Split a String by a Regex in JavaScript?
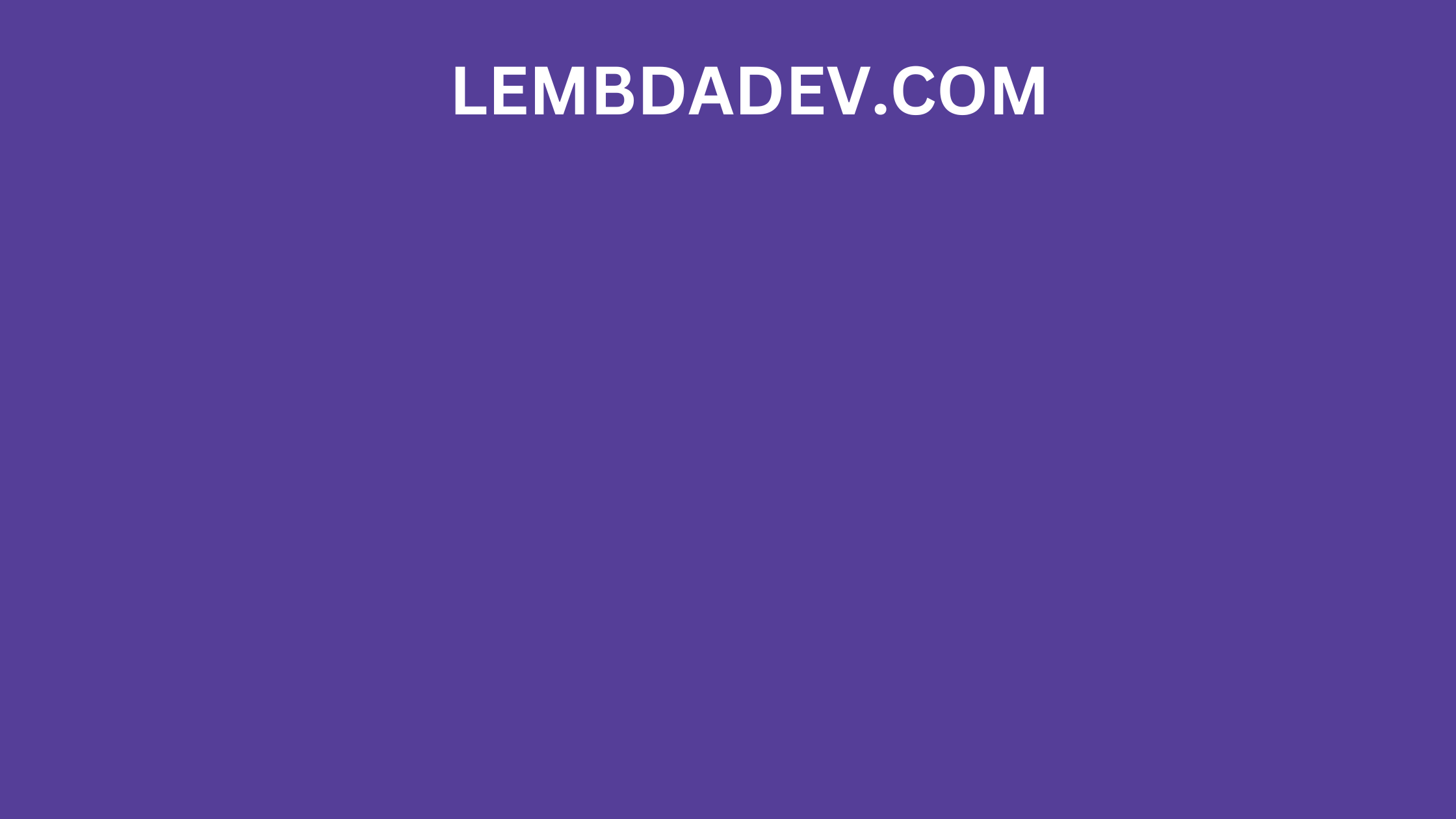
How to Split a String by a Regex in JavaScript?
How to Split a String by a Regex in JavaScript
JavaScript is a popular programming language that is used to create dynamic and interactive web pages. One common task in JavaScript is to split a string by a regular expression. This can be useful for a variety of purposes, such as parsing user input, processing text data, or extracting specific information from a string.
To split a string by a regular expression in JavaScript, you can use the string.split()
method. The string.split()
method splits a string into an array of substrings, using a specified separator. To split a string by a regular expression, you would pass the regular expression as the separator.
Example to Split a String by a Regex in JavaScript:
// Split a string by a regular expression
const string = "This is a string with spaces.";
const regex = /\s+/;
const words = string.split(regex);
console.log(words); // ['This', 'is', 'a', 'string', 'with', 'spaces.']
In this example, the regular expression /\s+/
matches any whitespace character, including spaces, tabs, and newlines. This means that the string.split()
method will split the string into an array of words, separated by whitespace characters.
You can use regular expressions to split a string by any pattern, not just whitespace characters. For example, you could split a string by commas, semicolons, or any other delimiter. You could also split a string by a specific pattern of characters, such as email addresses or phone numbers.
Example:
// Split a string by commas
const string = 'This,is,a,string,with,commas.';
const regex = /,/;
const words = string.split(regex);
console.log(words); // ['This', 'is', 'a', 'string', 'with', 'commas']
// Split a string by email addresses
const string = 'This email address: john.doe@example.com is valid.';
const regex = /\b[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}\b/;
const emailAddresses = string.split(regex);
console.log(emailAddresses); // ['john.doe@example.com']
Conclusion
Splitting a string by a regular expression in JavaScript is a powerful and flexible way to parse text data. By using regular expressions, you can split a string by any pattern, not just whitespace characters. This can be useful for a variety of purposes, such as parsing user input, processing text data, or extracting specific information from a string.