- Published on
Google Interview Question(Compare Two JSON Object in Javascript)
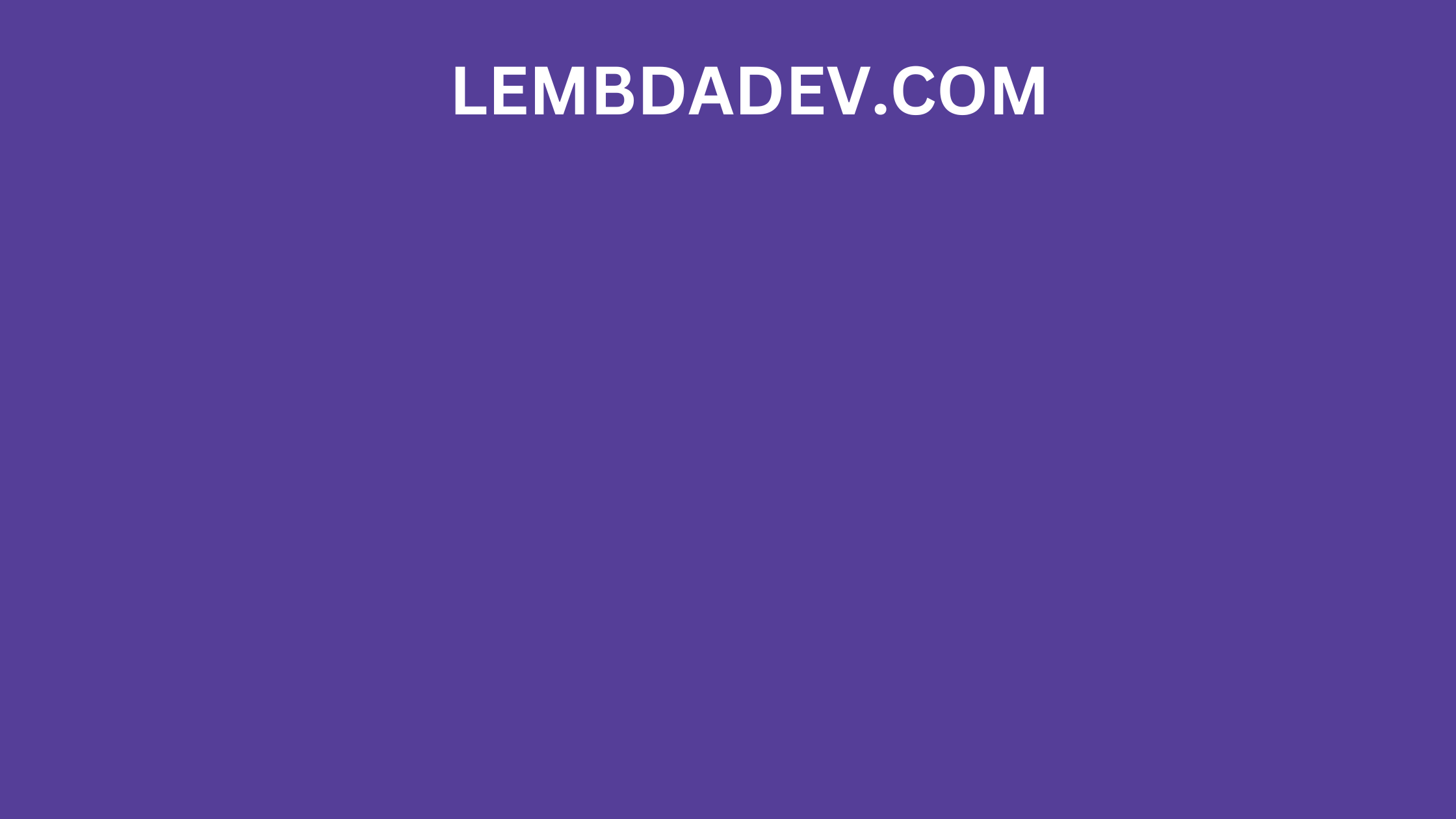
Google Interview Question(Compare Two JSON Object in Javascript)
Google Interview Question(Compare Two JSON Object in Javascript)
Question: Suppose you have two JSON "doc1" and "doc2". doc2 json is created by modifiying the doc1 JSON.
Return the difference between the doc1 and doc2.
Solution
You can use the following JavaScript function to find the difference between two JSON objects doc1
and doc2
:
function findJsonDifference(doc1, doc2) {
const diff = {};
for (const key in doc1) {
if (typeof doc2[key] === "undefined") {
diff[key] = doc1[key];
} else if (typeof doc1[key] === "object" && typeof doc2[key] === "object") {
const nestedDiff = findJsonDifference(doc1[key], doc2[key]);
if (Object.keys(nestedDiff).length > 0) {
diff[key] = nestedDiff;
}
} else if (doc1[key] !== doc2[key]) {
diff[key] = doc1[key];
}
}
for (const key in doc2) {
if (typeof doc1[key] === "undefined") {
diff[key] = doc2[key];
}
}
return diff;
}
// Example usage:
const doc1 = {
name: "John",
age: 30,
address: {
city: "New York",
zip: 10001,
},
};
const doc2 = {
name: "John",
age: 35,
address: {
city: "Los Angeles",
zip: 90001,
},
};
const difference = findJsonDifference(doc1, doc2);
console.log(difference);
This function recursively iterates through the keys of doc1
and compares them with the corresponding keys in doc2
. If a key exists in doc1
but not in doc2
, or if the values associated with a key are different between the two documents, it adds the key and value from doc1
to the diff
object. If the value of a key is an object, it recursively compares the nested objects. Finally, it also checks for keys that exist in doc2
but not in doc1
and adds those to the diff
object as well.
Additional Question:
Once you identify the difference. Create a separate function pass the difference and doc1, add both of them to create the doc2.
Solution
You can create a function called mergeJson
to merge the difference
object with doc1
to create doc2
. Here's how you can do it:
function mergeJson(doc1, difference) {
const doc2 = { ...doc1 }; // Create a shallow copy of doc1
for (const key in difference) {
if (typeof difference[key] === "object" && typeof doc2[key] === "object") {
doc2[key] = mergeJson(doc2[key], difference[key]);
} else {
doc2[key] = difference[key];
}
}
return doc2;
}
// Example usage:
const doc1 = {
name: "John",
age: 30,
address: {
city: "New York",
zip: 10001,
},
};
const difference = {
age: 35,
address: {
city: "Los Angeles",
zip: 90001,
},
};
const doc2 = mergeJson(doc1, difference);
console.log(doc2);
This mergeJson
function iterates through the keys of the difference
object. If a key in difference
has an object value and the corresponding key in doc2
is also an object, it recursively merges the objects. Otherwise, it simply assigns the value from difference
to doc2
. Finally, it returns doc2
, which now represents the modified version of doc1
according to the differences specified in the difference
object.