- Published on
How to Clear Text Selection using JavaScript?
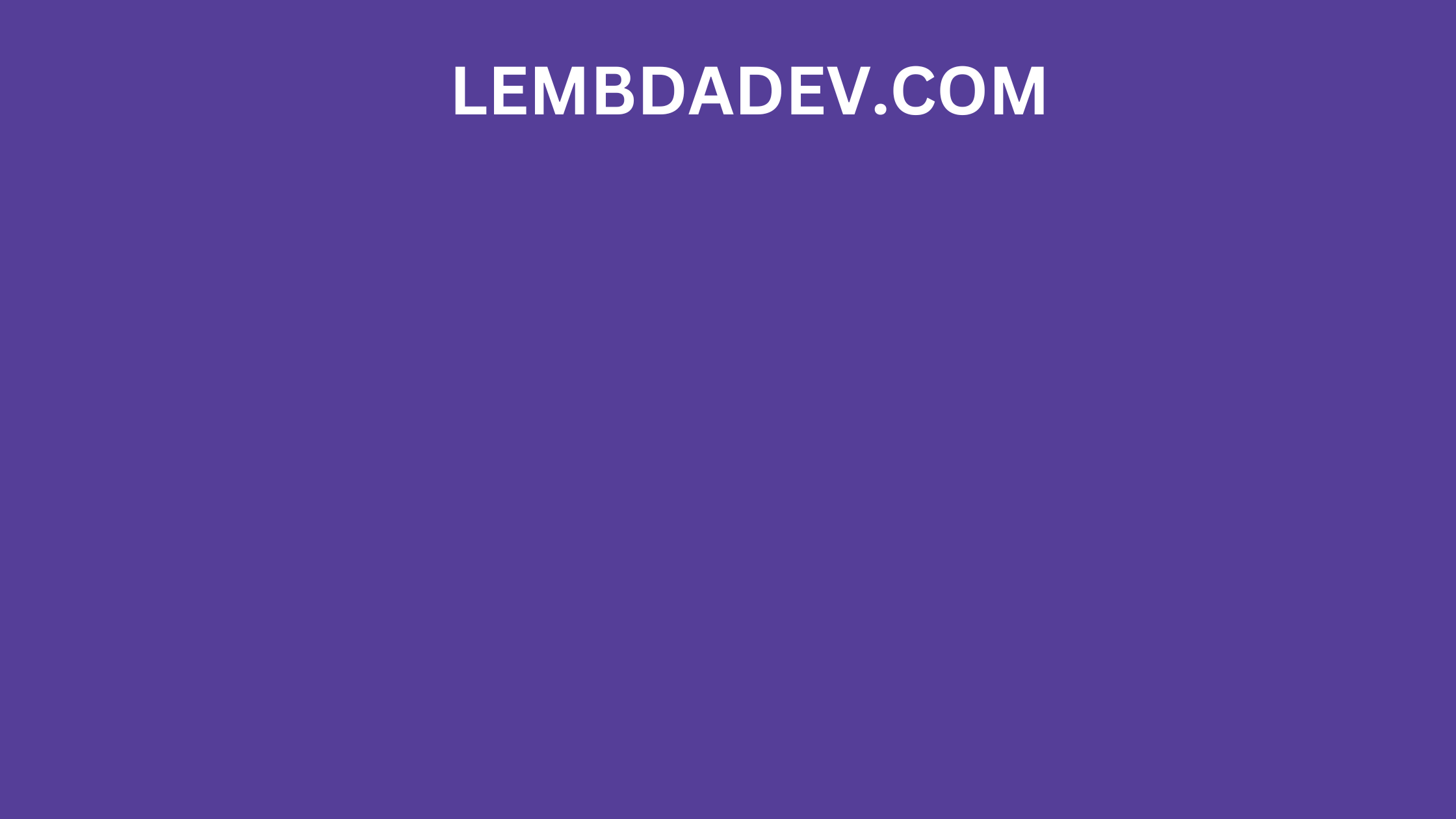
How to Clear Text Selection using JavaScript?
How to Clear Text Selection using JavaScript
JavaScript is a powerful language that can be used to manipulate web pages in a variety of ways. One common task is to clear text selection. This can be useful for a variety of purposes, such as preventing users from accidentally selecting text, or clearing the selection after a user has performed an action.
There are two main ways to clear text selection using JavaScript:
Use the
window.getSelection()
method. Thewindow.getSelection()
method returns aSelection
object, which represents the current text selection. To clear the selection, you can call theremoveAllRanges()
method on theSelection
object.Use the
document.execCommand()
method. Thedocument.execCommand()
method can be used to execute a variety of commands on the document, including clearing the text selection. To clear the selection, you would call thedocument.execCommand()
method with theunselect
command.
Examples:
// Clear text selection using the window.getSelection() method
const selection = window.getSelection();
selection.removeAllRanges();
// Clear text selection using the document.execCommand() method
document.execCommand("unselect");
Which method should I use to clear text selection?
The window.getSelection()
method is generally considered to be the better method for clearing text selection. This is because it is more explicit and less likely to cause unexpected results.
Conclusion
Clearing text selection using JavaScript is a simple and straightforward process. By following the steps above, you can easily clear the text selection on any web page.