- Published on
JavaScript array/list comprehensions?
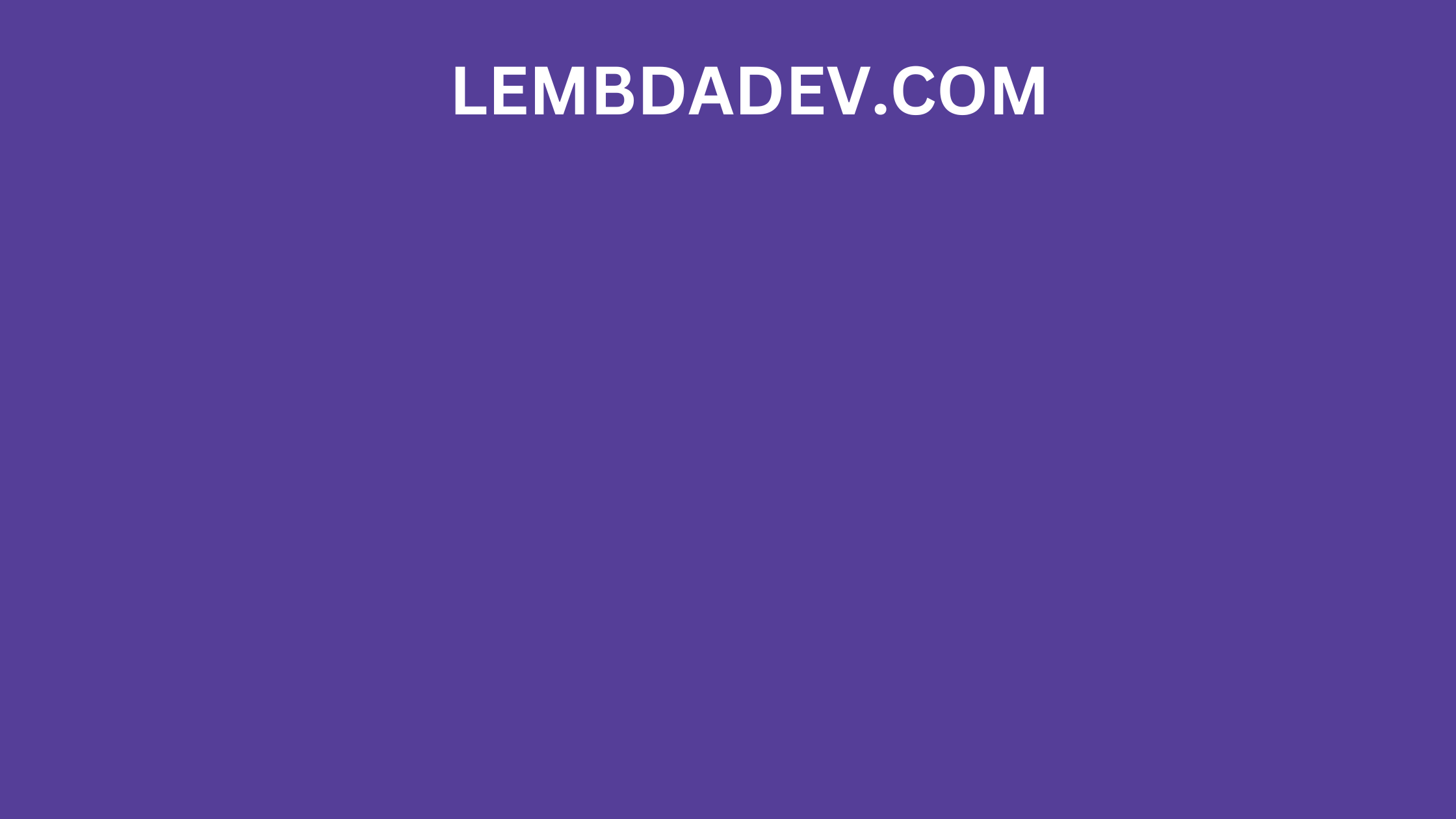
JavaScript array/list comprehensions?
JavaScript array/list comprehensions
JavaScript array/list comprehensions are a concise way to create a new array from an existing array. They are similar to Python list comprehensions, but there are some important differences.
How to create an array comprehension in JavaScript?
To create an array comprehension in JavaScript, you use the following syntax:
[<expression> for (<element> in <array>) if (<condition>)]
The <expression>
can be any JavaScript expression. The <element>
is the current element in the <array>
. The <condition>
is an optional condition that must be true for the element to be included in the new array.
Here is an example of a simple array comprehension:
const numbers = [1, 2, 3, 4, 5];
// Create a new array containing the squares of the numbers in the `numbers` array.
const squares = [number * number for (number in numbers)];
console.log(squares); // [1, 4, 9, 16, 25]
This code will create a new array called squares
that contains the squares of the numbers in the numbers
array.
- Array comprehensions can also be used to filter the elements of an array. For example, the following code will create a new array containing only the even numbers in the
numbers
array:
const evenNumbers = [number for (number in numbers) if (number % 2 === 0)];
console.log(evenNumbers); // [2, 4]
- Array comprehensions can also be nested. For example, the following code will create a new array containing all of the possible pairs of numbers in the
numbers
array:
const pairs = [[number1, number2] for (number1 in numbers) for (number2 in numbers) if (number1 !== number2)];
console.log(pairs); // [[1, 2], [1, 3], [1, 4], [1, 5], [2, 3], [2, 4], [2, 5], [3, 4], [3, 5], [4, 5]]
- Array comprehensions are a powerful tool for creating and manipulating arrays in JavaScript. They can be used to concisely and efficiently perform a variety of tasks, such as filtering, mapping, and combining arrays.
Here are some more examples of array comprehensions in JavaScript:
// Create a new array containing all of the vowels in the string "hello".
const vowels = ["aeiou"].includes(letter) for (letter in "hello");
console.log(vowels); // ["e", "o"]
// Create a new array containing all of the users in the `users` array who are over the age of 18.
const adultUsers = [user for (user in users) if (user.age > 18)];
console.log(adultUsers); // [user1, user2, ...]
// Create a new array containing all of the products in the `products` array that have a price greater than $100.
const expensiveProducts = [product for (product in products) if (product.price > 100)];
console.log(expensiveProducts); // [product1, product2, ...]
Conclusion
Array comprehensions can be a bit tricky to understand at first, but they are a powerful tool that can save you a lot of time and code. If you are working with arrays in JavaScript, I recommend learning how to use array comprehensions.