- Published on
useList Hook React
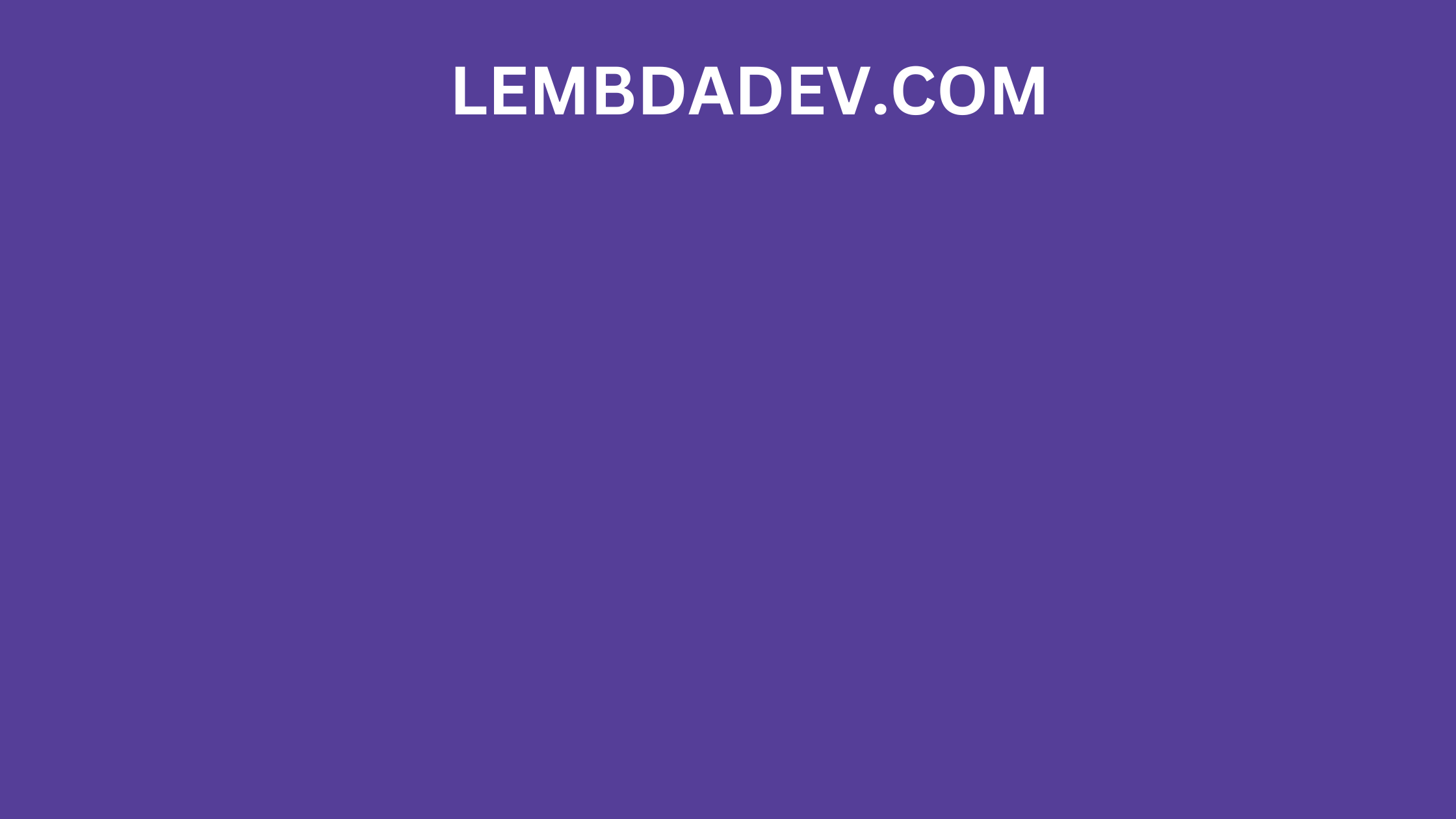
useList Hook React
useList Code and Usage
useList is a hook to manage a list of items.
import { useState } from "react";
const useList = (initialList = []) => {
const [list, setList] = useState(initialList);
const addItem = (item) => {
setList((prevList) => [...prevList, item]);
};
const removeItem = (index) => {
setList((prevList) => {
const newList = [...prevList];
newList.splice(index, 1);
return newList;
});
};
return {
list,
addItem,
removeItem,
};
};
// Usage
const ListExample = () => {
const { list, addItem, removeItem } = useList(["item1", "item2"]);
return (
<div>
<ul>
{list.map((item, index) => (
<li key={index}>
{item}
<button onClick={() => removeItem(index)}>Remove</button>
</li>
))}
</ul>
<button onClick={() => addItem("new item")}>Add Item</button>
</div>
);
};