- Published on
useGeolocation Hook React
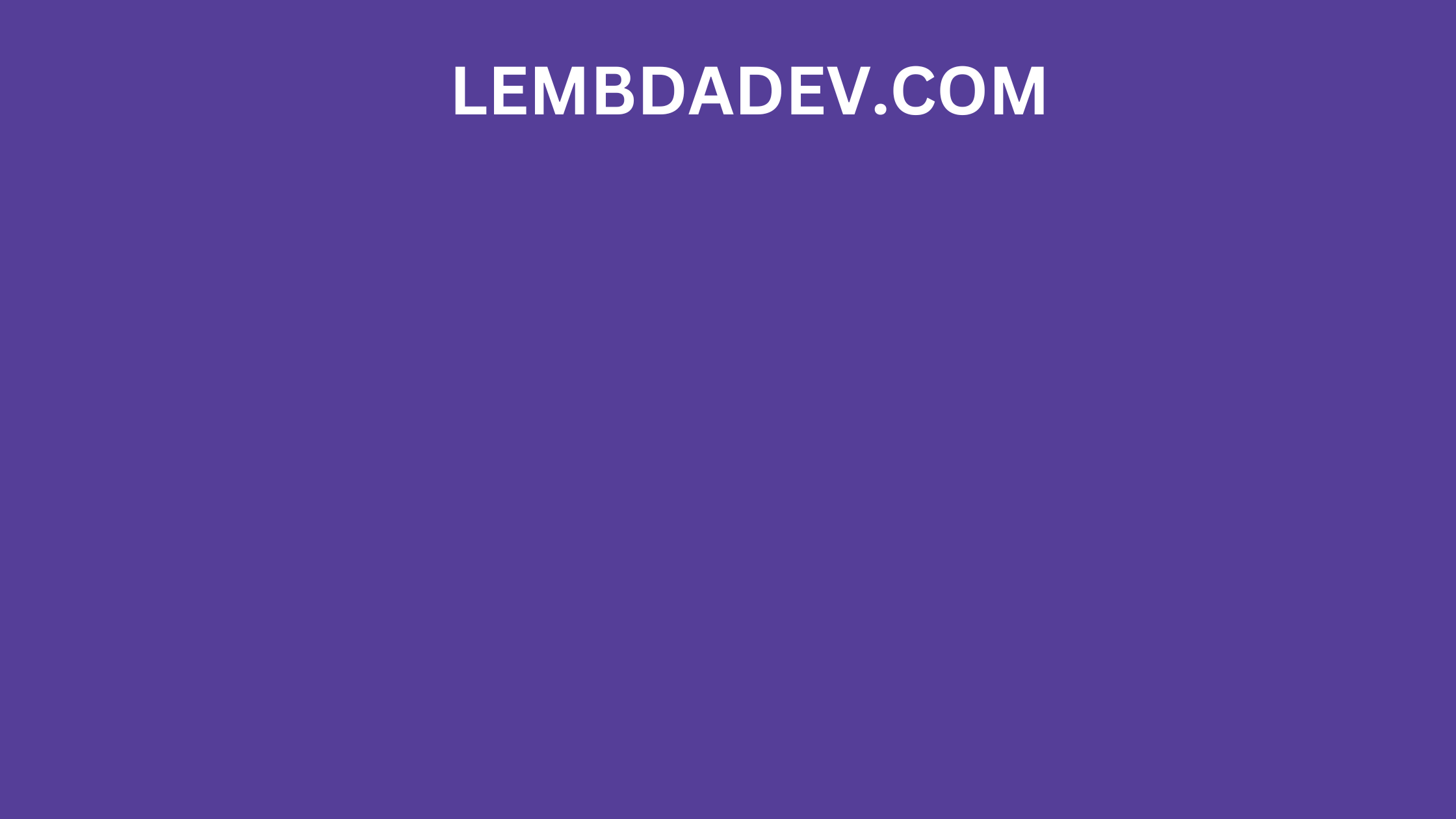
useGeolocation Hook React
useGeolocation Code and Usage
useGeolocation is a hook to access the user's geolocation.
import { useState, useEffect } from "react";
const useGeolocation = () => {
const [position, setPosition] = useState(null);
useEffect(() => {
const success = (pos) => {
const { latitude, longitude } = pos.coords;
setPosition({ latitude, longitude });
};
const error = (err) => {
console.error("Geolocation error:", err);
};
const options = {
enableHighAccuracy: true,
timeout: 10000,
maximumAge: 0,
};
const watchId = navigator.geolocation.watchPosition(
success,
error,
options
);
return () => {
navigator.geolocation.clearWatch(watchId);
};
}, []);
return position;
};
// Usage
const GeolocationExample = () => {
const position = useGeolocation();
if (!position) {
return <p>Geolocation not available.</p>;
}
return (
<div>
<p>Latitude: {position.latitude}</p>
<p>Longitude: {position.longitude}</p>
</div>
);
};