- Published on
Memoization in javascript
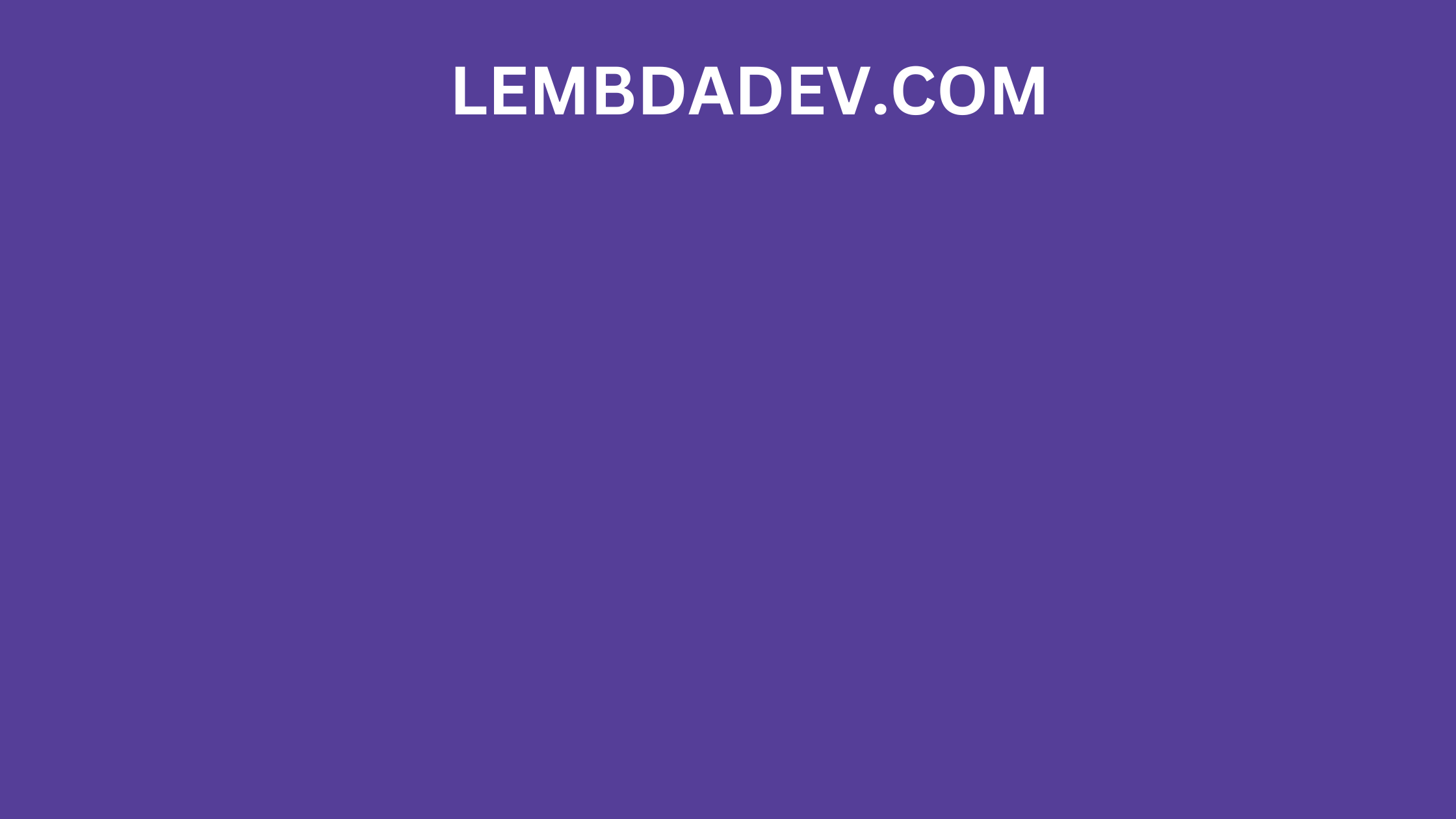
Memoization in javascript
Memoization in JavaScript
What is memoization?
Memoization is a programming technique that stores the results of expensive function calls and returns the cached result when the same inputs are used again. This can improve the performance of applications by avoiding redundant computations.
How does memoization work?
Memoization works by caching the results of function calls. When a function is called with a given set of inputs, the result of the function call is stored in a cache. The next time the function is called with the same set of inputs, the result is retrieved from the cache and returned, without having to recalculate the function again.
Benefits of memoization
There are a number of benefits to using memoization, including:
- Improved performance: Memoization can significantly improve the performance of applications by avoiding redundant computations. This is especially beneficial for applications that make a lot of expensive function calls.
- Reduced memory usage: Memoization can reduce the memory usage of applications by caching the results of function calls. This is because the results of function calls do not need to be recalculated each time the function is called.
- Simpler code: Memoization can make code simpler and easier to maintain. This is because memoization can eliminate the need to write code to check if a function's result has already been calculated.
Drawbacks of memoization
There are also a few drawbacks to using memoization, including:
- Increased memory usage: Memoization can increase the memory usage of applications by caching the results of function calls. However, this drawback is often offset by the performance improvements that memoization can provide.
- Debugging difficulties: Memoization can make code more difficult to debug. This is because it can be difficult to track down the source of a bug when the results of function calls are being cached.
How to use memoization in JavaScript
There are a few different ways to use memoization in JavaScript. One common approach is to use a closure to store the cache. The following code shows an example of how to use a closure to implement memoization:
function memoize(fn) {
const cache = {};
return (...args) => {
const key = args.join(",");
if (cache[key]) {
return cache[key];
}
const result = fn(...args);
cache[key] = result;
return result;
};
}
const memoizedFibonacci = memoize(function fibonacci(n) {
if (n < 2) {
return n;
}
return fibonacci(n - 1) + fibonacci(n - 2);
});
console.log(memoizedFibonacci(10)); // 55
console.log(memoizedFibonacci(10)); // 55
In this example, the memoize()
function takes a function as an argument and returns a memoized version of that function. The memoized function caches the results of its function calls and returns the cached result when the same inputs are used again.
Another approach to using memoization in JavaScript is to use a library that implements memoization. There are a number of different libraries that implement memoization, such as memoize-one
and lodash.memoize
.
Real-world examples of memoization
Memoization is used in a variety of real-world applications, including:
- Web development: Browsers use memoization to cache the results of function calls that are needed to download resources for a web page. This can improve the performance of web pages by avoiding the need to download the same resources multiple times.
- Data structures: Memoization is used in some data structures, such as hash tables and trie trees, to improve their performance.
- Machine learning: Memoization is used in some machine learning algorithms, such as dynamic programming algorithms, to improve their performance.
Conclusion
Memoization is a powerful technique that can improve the performance of applications by avoiding redundant computations. It is important to be aware of the benefits and drawbacks of memoization before using it in your code.