- Published on
How to access nested object, array in javascript?
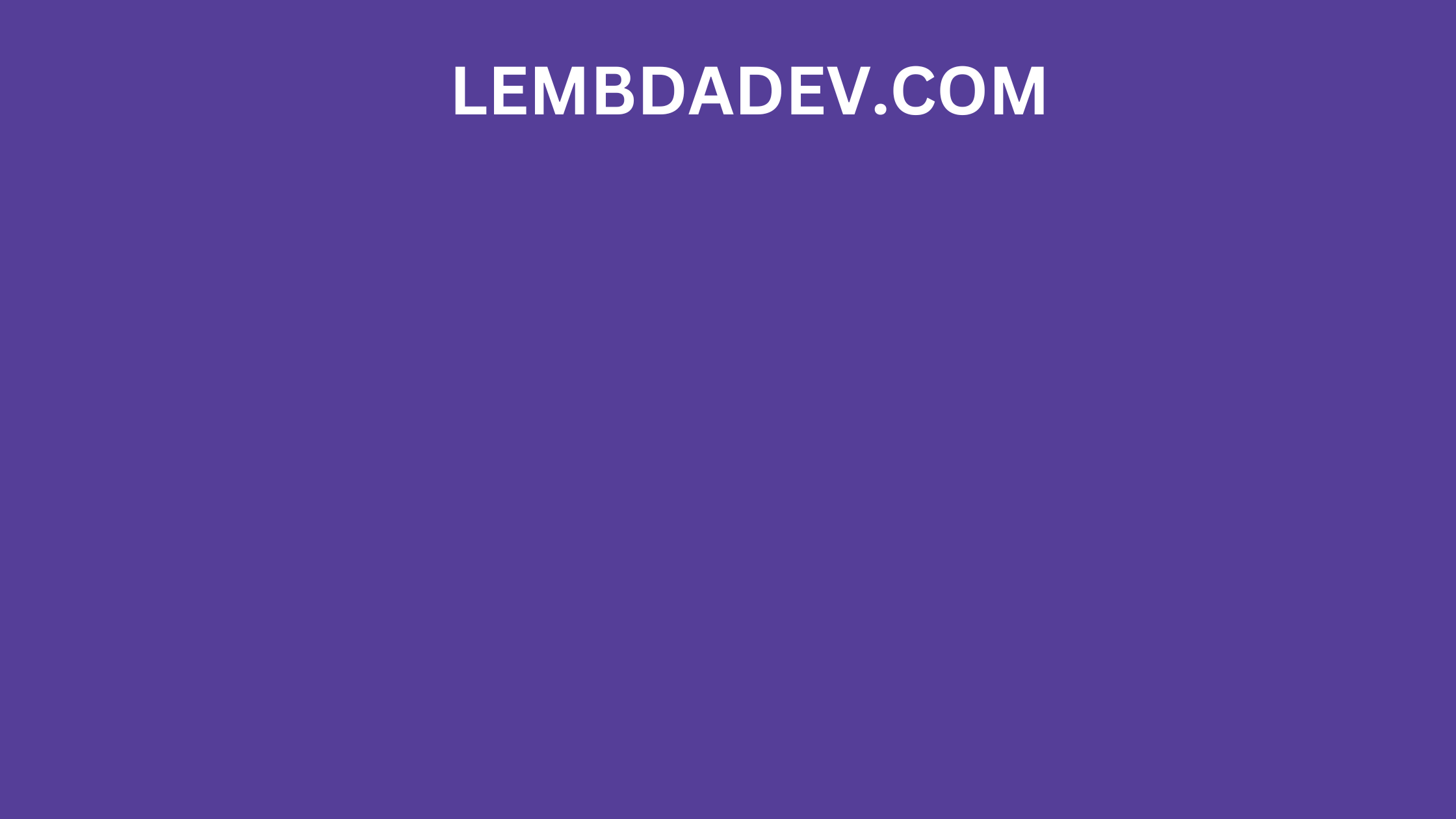
How to access nested object, array in javascript?
JavaScript: Access Nested Object
What is nested objects?
Let's take an example of nested object-
const userObj = {
id: 1,
info: {
age: 20
name:{
first_name: "John"
last_name: "Martin"
},
address: {
city: "Newyork",
state: ""
}
}
};
if we have to access user's first_name and city we can do like below -
first_name = userObj.info.name.first_name;
city = userObj.info.address.city;
This is very easy, we don't face any problem but if userObj
object doesn't have property then it will throw error
const userObj = {
id: 1,
info: {
address: {
city: "Newyork",
state: "",
},
},
};
const name = userObj.info.name.first_name; // Cannot read property 'first_name' of undefined
To avoid this we usually check property before accessing if that exist or not. This is fine for object with nested level 2 or 3, but when we have object with nested level 5 or 6 then the code become large and messy.
// This is fine
const age = userObj && userObj.info ? userObj.info.age : null;
// code become messy
const city =
userObj &&
userObj.info &&
userObj.info.address &&
userObj.info.address.primary
? userObj.info.address.primary.city
: null;
There are different ways to handle, we will discuss some of the best practice here -
Oliver Steele's Nested Object Access Pattern
Using this method you will never run to the error Cannot read property _ of undefined
and this is very straightforward
const name = ((((userObj || {}).info || {}).address || {}).primary || {}).city;
Access Nested Objects Using Array Reduce
Below method getNestedObject
can be used to access nested object without much complexity, you just need to pass object and path
const getNestedObject = (nestedObj, pathArr) => {
return pathArr.reduce(
(obj, key) => (obj && obj[key] !== "undefined" ? obj[key] : undefined),
nestedObj
);
};
// pass in your object structure as array elements
const name = getNestedObject(user, ["info", "address", "primary", "city"]);
How to access elements in nested arrays in javascript?
Nested array is nothing but multi-dimensional array or in simple words we can say array elements are also error.
The structure of nested array looks like this -
const Array=[[values,[nest value…],…],[values, [nest value…],….],[values, [nest value…],….]]
We can access nested element using without getting any error -
const a = [1, [2, [3, 4]], [5, 6]];
console.log(a[0]); // 1
console.log(a[1][0]); //2
console.log(a[1][1]); //[3,4]
console.log(a[1][1][0]); //3
console.log(a[1][0]); //undefined
console.log(a[1][0][0]); // Cannot read properties of undefined (reading '0')
Usually dev use for loop to find the value wchich is too complex, easy and straight forward way to do this written below -
// Let's say if we have to access value of arr[a][b][c][d][e]
const nestedValue = ((((arr[a] || [])[b] || [])[c] || [])[d || [])[e]
So we have discussed about accessing element in nested object and nested array, hope you find this helpful.