- Published on
5 ways to flatten an array in javascript
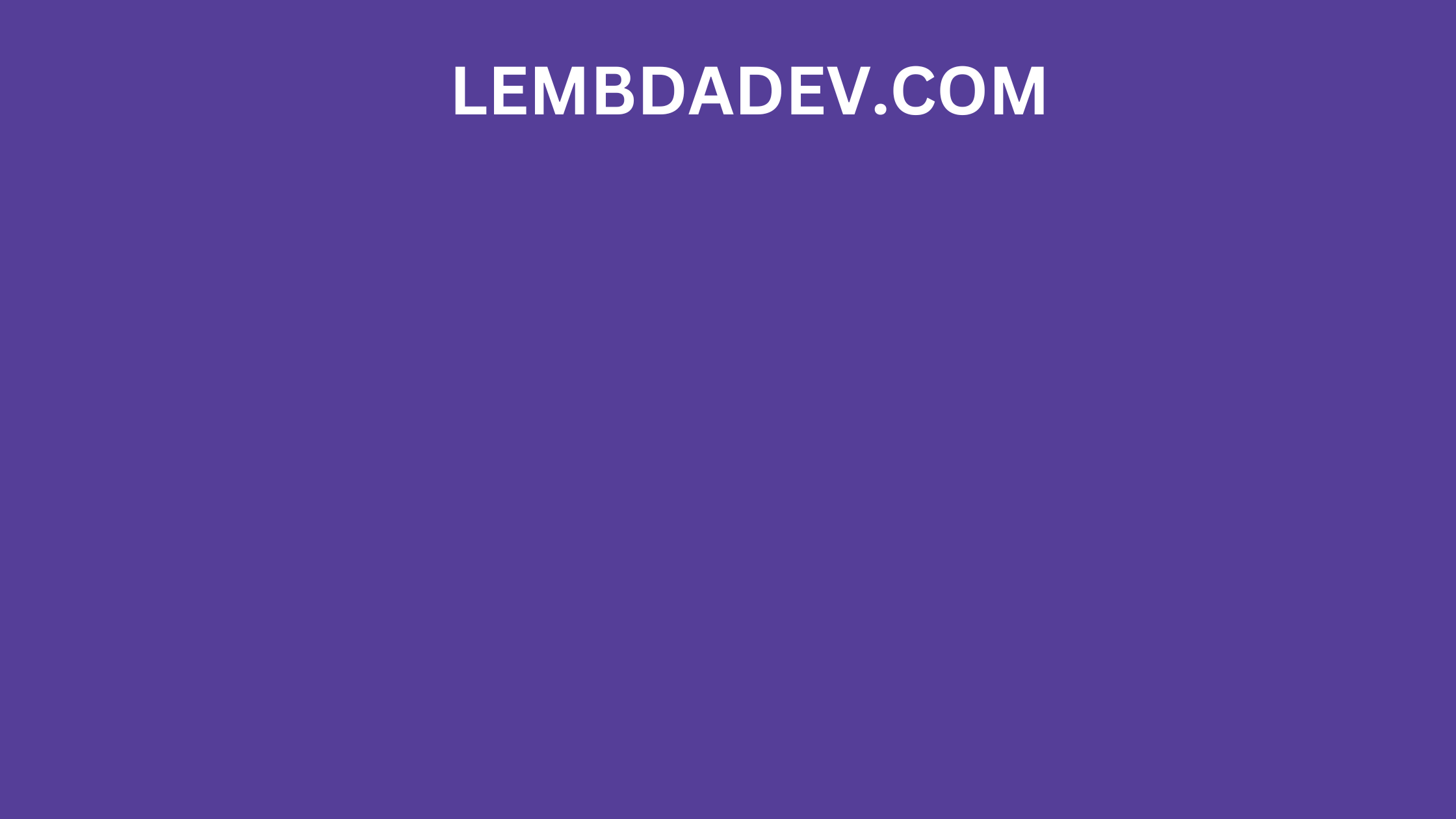
5 ways to flatten an array in javascript
What is Flattening of an array?
Flattening an array is a process of reducing the dimensionality of an array from multi dimensions to one dimension array.
Let's understand this using an example given below
// a multi dimensional array
const arr = [
[1, 2],
[3, 4, 5],
[5, 6, [7, 8]],
[9, 10, [11, 12]],
];
// After flattening array would looks like below
const flattenArr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12];
Different methods to flatten an array in javascript
1. Using concat() and spread operator :
In this case, when we apply the spread operator to the array arr that we previously declared, the Spread syntax () permits the concatenation operation to be applied to all elements of the array and stores the result in an empty array, giving us a flattened array.
let flatArray = [].concat(...arr);
//Output: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]
2. Using concat() and apply()
Here we are using concat() and apply() methods where we pass two arguments to the apply function which will be concated with empty array.
let flatArray = [].concat.apply([], arr);
//Output: [ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12 ]
3. Flatten array using the reduce method
With Reduce method you create a flatten
method which will execute recursively and flatten array.
let flatten = arr.reduce((acc, curVal) => {
return acc.concat(curVal);
}, []);
console.log(flatten(arr));
//Output: [ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]
4. Flatten array using stack
Using stack data structure you can flatten this array which will work non recursively.
function flatten(input) {
const stack = [...input];
const res = [];
while (stack.length) {
const next = stack.pop();
if (Array.isArray(next)) {
stack.push(...next);
} else {
res.push(next);
}
}
return res.reverse();
}
console.log(flatten(arr));
//Output: [ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]
5. Using Flat method :
ES2019 introduced the Array.prototype.flat()
method which you could use to flatten the arrays. the flat() method is used to create a new array with all sub-array elements concatenated into it recursively up to the specified depth. The depth parameter is optional and when no depth is specified, a depth of 1 is considered by default. To flatten complete array using flat
methood you can use array.flat(Infinity)
.
const arr = [
[1, 2],
[3, 4, 5],
[5, 6, [7, 8]],
[9, 10, [11, 12]],
];
const arr1 = arr.flat(1);
// output: [1, 2, 3, 4, 5, 5, 6, [7, 8], 9, 10, [11, 12]]
const flattenArr = array.flat(Infinity);
// output: [ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]
So these are the different way we can flatten an array using javascript.