- Published on
How Functions Work in JavaScript (Basics to Advanced)?
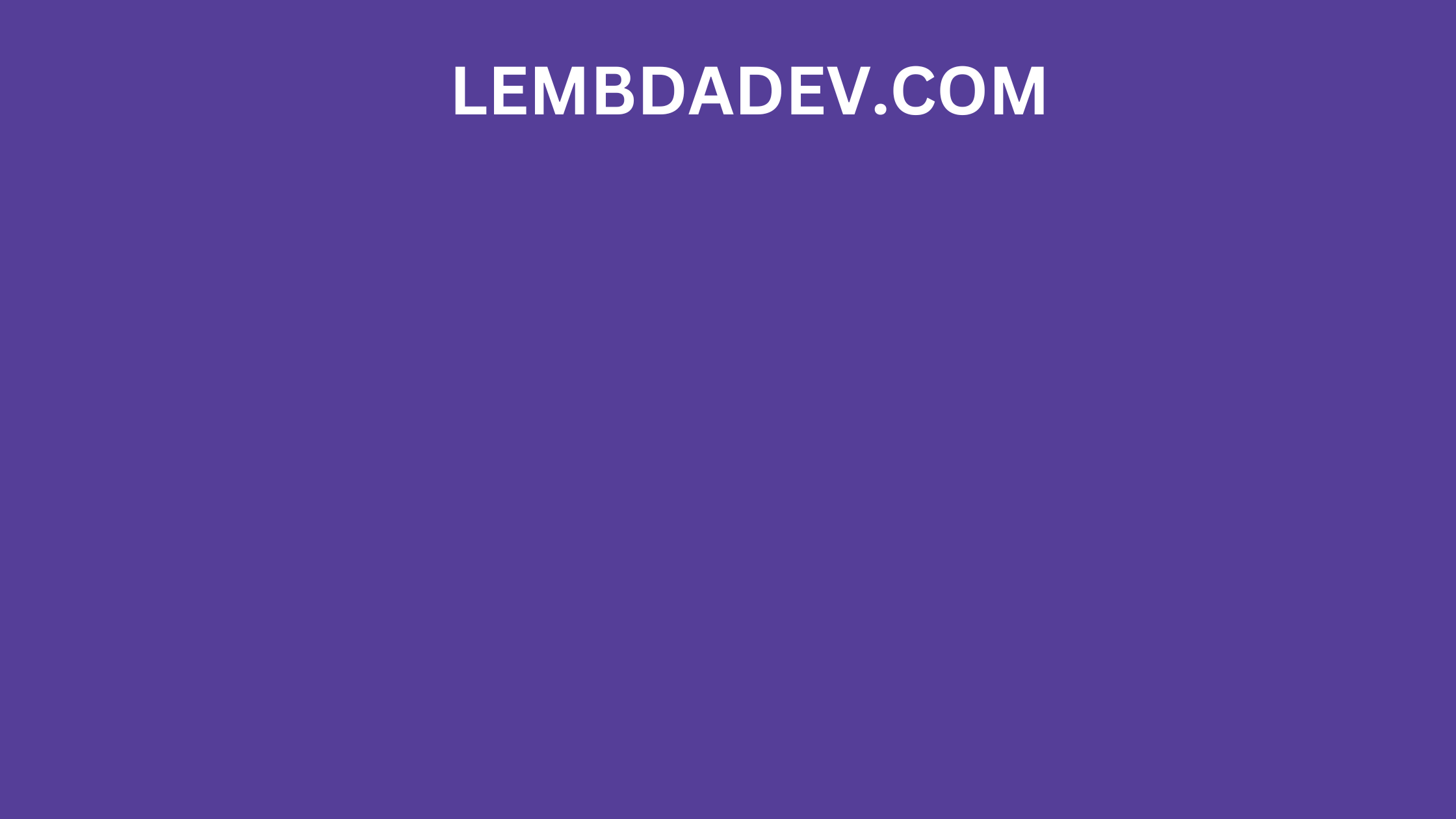
How Functions Work in JavaScript (Basics to Advanced)?
How Functions Work in JavaScript?
Functions are a fundamental part of JavaScript. They allow us to encapsulate code and reuse it throughout our program. Functions can also take parameters and return values, which makes them very powerful.
Define a function in JavaScript
To define a function in JavaScript, we use the function
keyword. The function name is followed by a list of parameters, enclosed in parentheses. The function body is enclosed in curly braces.
Here is an example of a simple function:
function greet(name) {
return `Hello, ${name}!`;
}
This function takes one parameter, name
, and returns a string greeting the person with that name.
Call a function in JavaScript
To call a function, we simply type the function name, followed by parentheses, and optionally any arguments we want to pass to the function.
For example, to call the greet()
function with the name "Bard", we would write the following:
const greeting = greet("Bard");
The variable greeting
would then contain the string "Hello, Bard!".
Types of Functions in JavaScript
There are two main types of functions in JavaScript: named functions and anonymous functions.
1. Named functions
Named functions are defined using the function
keyword, followed by the function name and a list of parameters, enclosed in parentheses. The function body is enclosed in curly braces.
function greet(name) {
return `Hello, ${name}!`;
}
2. Anonymous functions
Anonymous functions are defined without a name, and can only be called by passing them as an argument to another function.
const add = function (a, b) {
return a + b;
};
In addition to these two main types of functions, there are also a number of other types of functions in JavaScript, including:
The IIFT function: The IIFT function, or Immediately Invoked Function Expression, is a powerful tool in JavaScript that can be used for a variety of purposes. It is a function that is defined and executed immediately, without having to be explicitly called. This is done by enclosing the function in parentheses.**
Arrow functions: Arrow functions are a concise way to write function expressions. They are similar to function expressions, but they use a shorter syntax.
const greet = (name) => `Hello, ${name}!`;
- Generator functions: Generator functions are a special type of function that can be used to pause and resume execution. They are useful for implementing asynchronous code. Generator Function in JavaScript detailed guide
function* generateNumbers() {
for (let i = 1; i <= 10; i++) {
yield i;
}
}
const numbers = generateNumbers();
console.log(numbers.next().value); // Outputs 1
console.log(numbers.next().value); // Outputs 2
console.log(numbers.next().value); // Outputs 3
- Async functions: Async functions are a special type of function that can be used to write asynchronous code in a more concise and readable way.
async function fetchData() {
const response = await fetch("https://example.com/api/data");
return await response.json();
}
const data = await fetchData();
- Closure Function: A closure is the combination of a function bundled together (enclosed) with references to its surrounding state (the lexical environment). In other words, a closure gives you access to an outer function's scope from an inner function.
How closure works in javascript?
Examples of Functions in JavaScript
Here are some examples of how to use functions in JavaScript:
// Define a function that greets the user by name
function greet(name) {
return `Hello, ${name}!`;
}
// Call the function and pass in the user's name
const greeting = greet("Bard");
// Log the greeting to the console
console.log(greeting); // Outputs "Hello, Bard!"
// Define a function that adds two numbers
function add(a, b) {
return a + b;
}
// Call the function and pass in two numbers
const sum = add(1, 2);
// Log the sum to the console
console.log(sum); // Outputs 3
// Define an arrow function that greets the user by name
const greet = (name) => `Hello, ${name}!`;
// Call the arrow function and pass in the user's name
const greeting = greet("Bard");
// Log the greeting to the console
console.log(greeting); // Outputs "Hello, Bard!"
// Define a generator function that generates a sequence of numbers
function* generateNumbers() {
for (let i = 1; i <= 10; i++) {
yield i;
}
}
// Call the generator function and get the next number in the sequence
const nextNumber = generateNumbers().next().value;
// Log the next number to the console
console.log(nextNumber); // Outputs 1
// Define an async function that fetches data from an API
async function fetchData() {
const response = await fetch("https://example.com/api/data");
return await response.json();
}
// Call the async function and get the data from the API
const data = await fetchData();
// Log the data to the console
console.log(data); // Outputs the data from the API
Benefits of Using Functions in JavaScript
There are a number of benefits to using functions in JavaScript, including:
- Code reuse: Functions can be reused throughout your code, which can save you time and effort.
- Modularity: Functions can be used to modularize your code, making it easier to understand and maintain.
- Abstraction: Functions can be used to abstract away complex logic, making your code more readable and maintainable.
- Performance: Functions can improve the performance of your code by caching results and avoiding unnecessary recalculations.
Conclusion
Functions are a powerful tool that can help you write more organized, reusable, efficient, and performant JavaScript code. By learning how to use functions effectively, you can become a better JavaScript developer.