- Published on
useKeypres Hook React
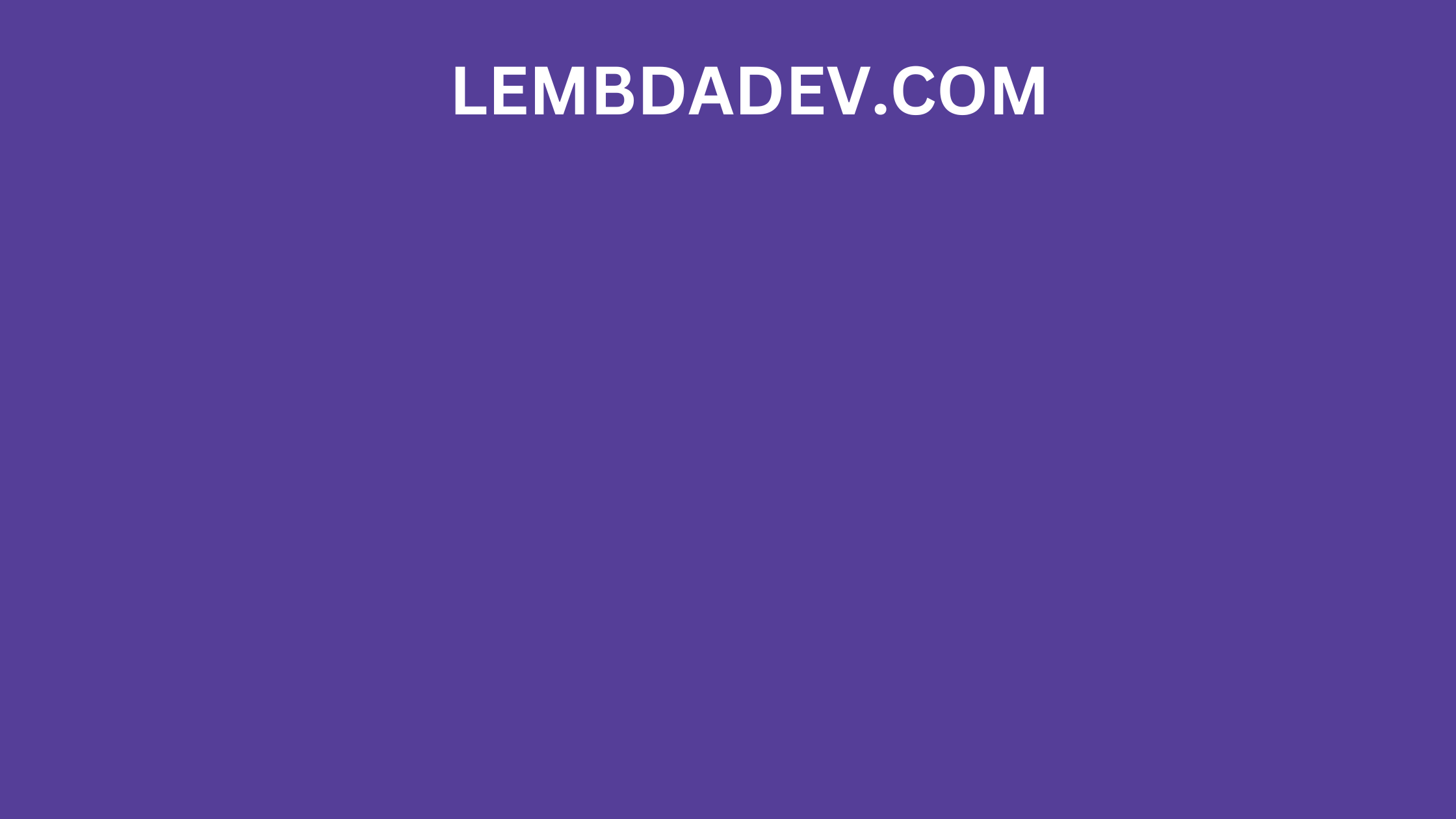
useKeypres Hook React
useKeypres Code and Usage
useKeypres is a hook to detect key presses.
import { useEffect } from "react";
const useKeypress = (targetKey, callback) => {
useEffect(() => {
const handleKeyPress = (event) => {
if (event.key === targetKey) {
callback();
}
};
document.addEventListener("keydown", handleKeyPress);
return () => {
document.removeEventListener("keydown", handleKeyPress);
};
}, [targetKey, callback]);
};
// Usage
const KeypressExample = () => {
const handleKeyPress = () => {
console.log("Enter key pressed.");
};
useKeypress("Enter", handleKeyPress);
return <div>Press the Enter key.</div>;
};