- Published on
useFetch Hook React
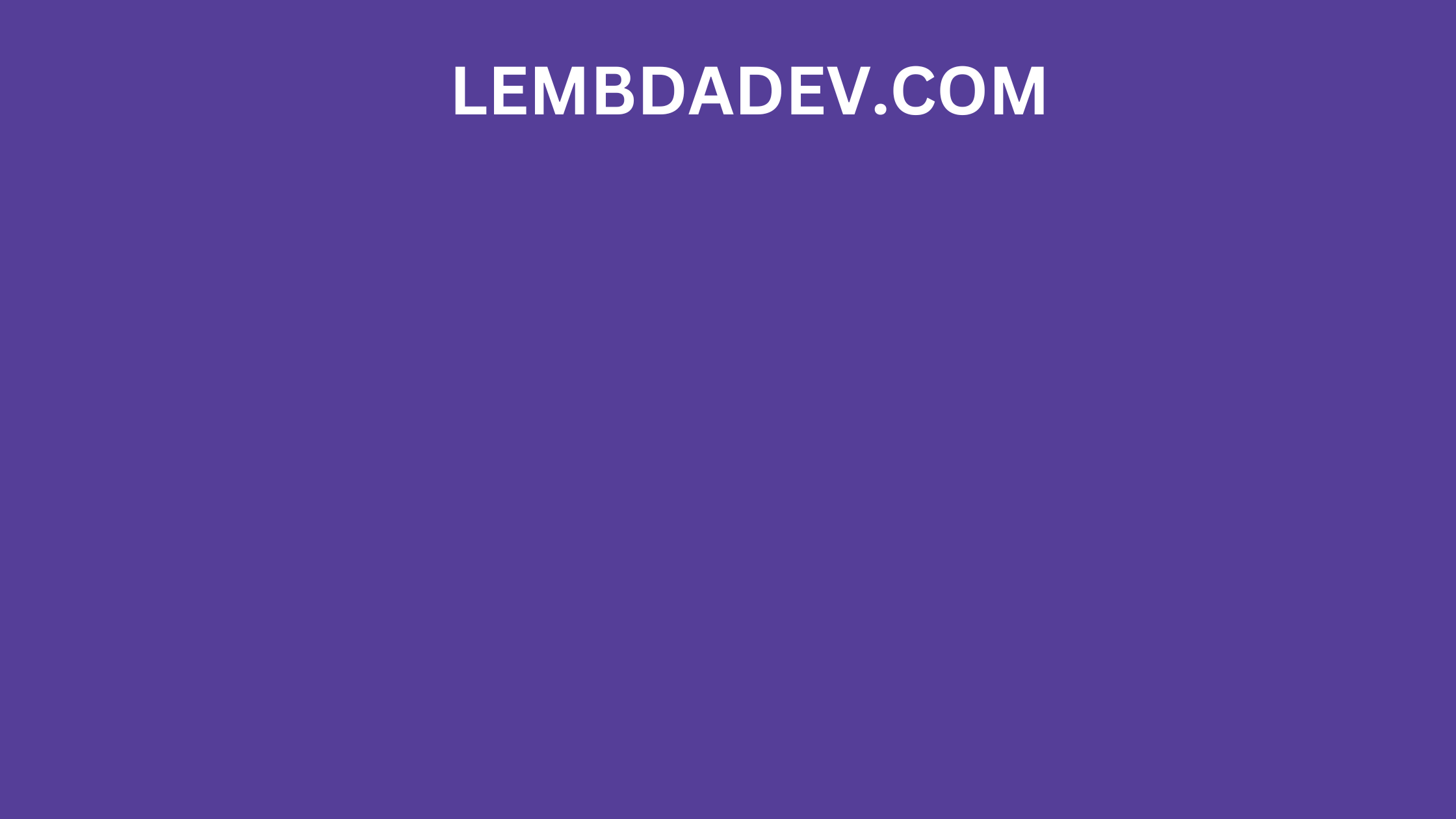
useFetch Hook React
useFetch Code and Usage
useFetch is a hook to make HTTP requests.
import { useState, useEffect } from "react";
const useFetch = (url) => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(url);
const json = await response.json();
setData(json);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
};
fetchData();
}, [url]);
return { data, loading, error };
};
// Usage
const FetchExample = () => {
const { data, loading, error } = useFetch(
"https://jsonplaceholder.typicode.com/posts/1"
);
if (loading) {
return <p>Loading...</p>;
}
if (error) {
return <p>Error: {error.message}</p>;
}
return (
<div>
<p>Title: {data.title}</p>
<p>Body: {data.body}</p>
</div>
);
};