- Published on
useCountdown Hook React
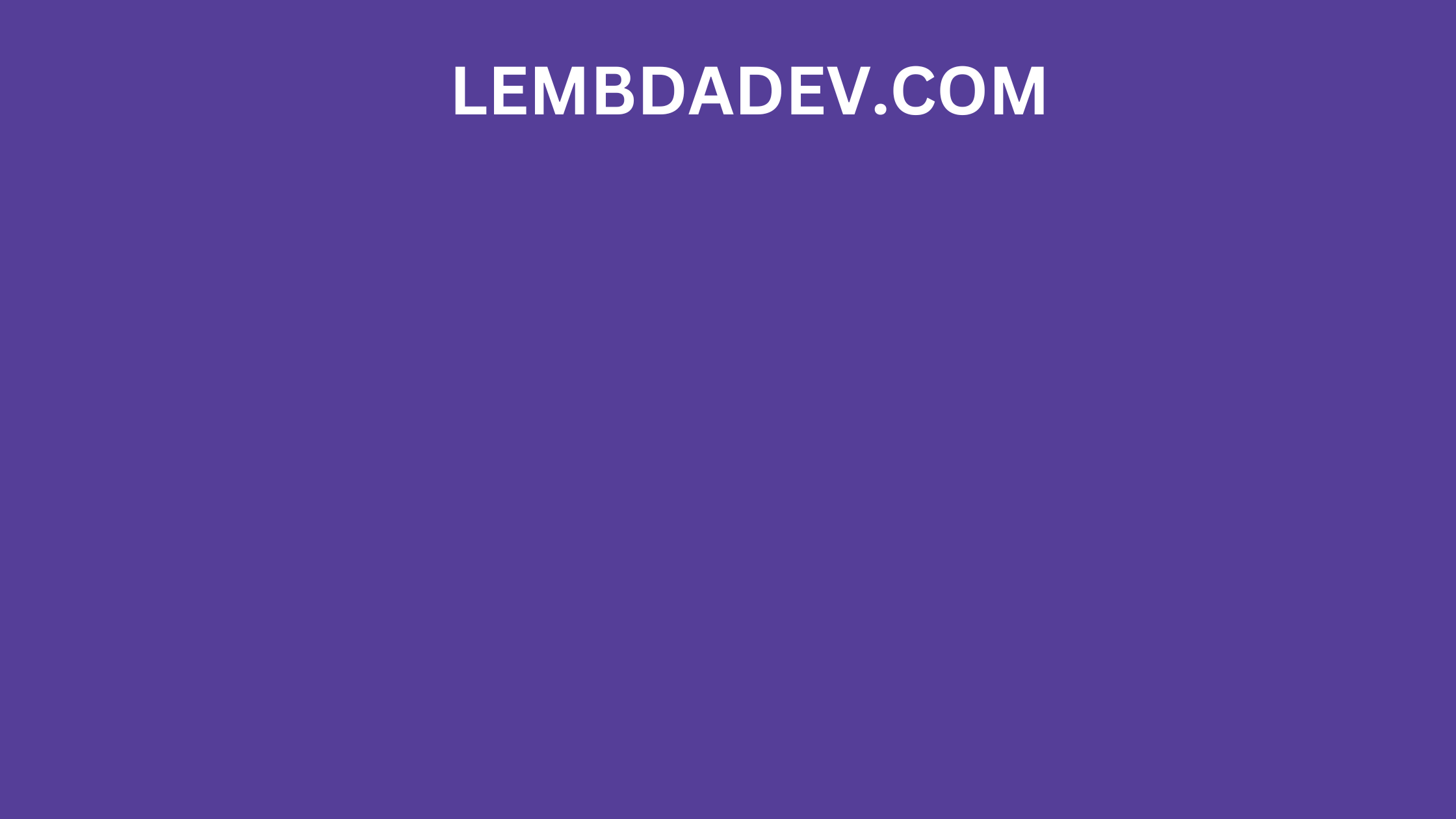
useCountdown Hook React
useCountdown Code and Usage
useCountdown is a hook to implement a countdown timer.
import { useState, useEffect } from "react";
const useCountdown = (initialTime) => {
const [time, setTime] = useState(initialTime);
useEffect(() => {
if (time > 0) {
const timer = setInterval(() => {
setTime((prevTime) => prevTime - 1);
}, 1000);
return () => {
clearInterval(timer);
};
}
}, [time]);
return time;
};
// Usage
const CountdownExample = () => {
const countdownTime = useCountdown(10);
return (
<div>
<p>Countdown: {countdownTime}</p>
</div>
);
};