- Published on
How to create a style tag using JavaScript?
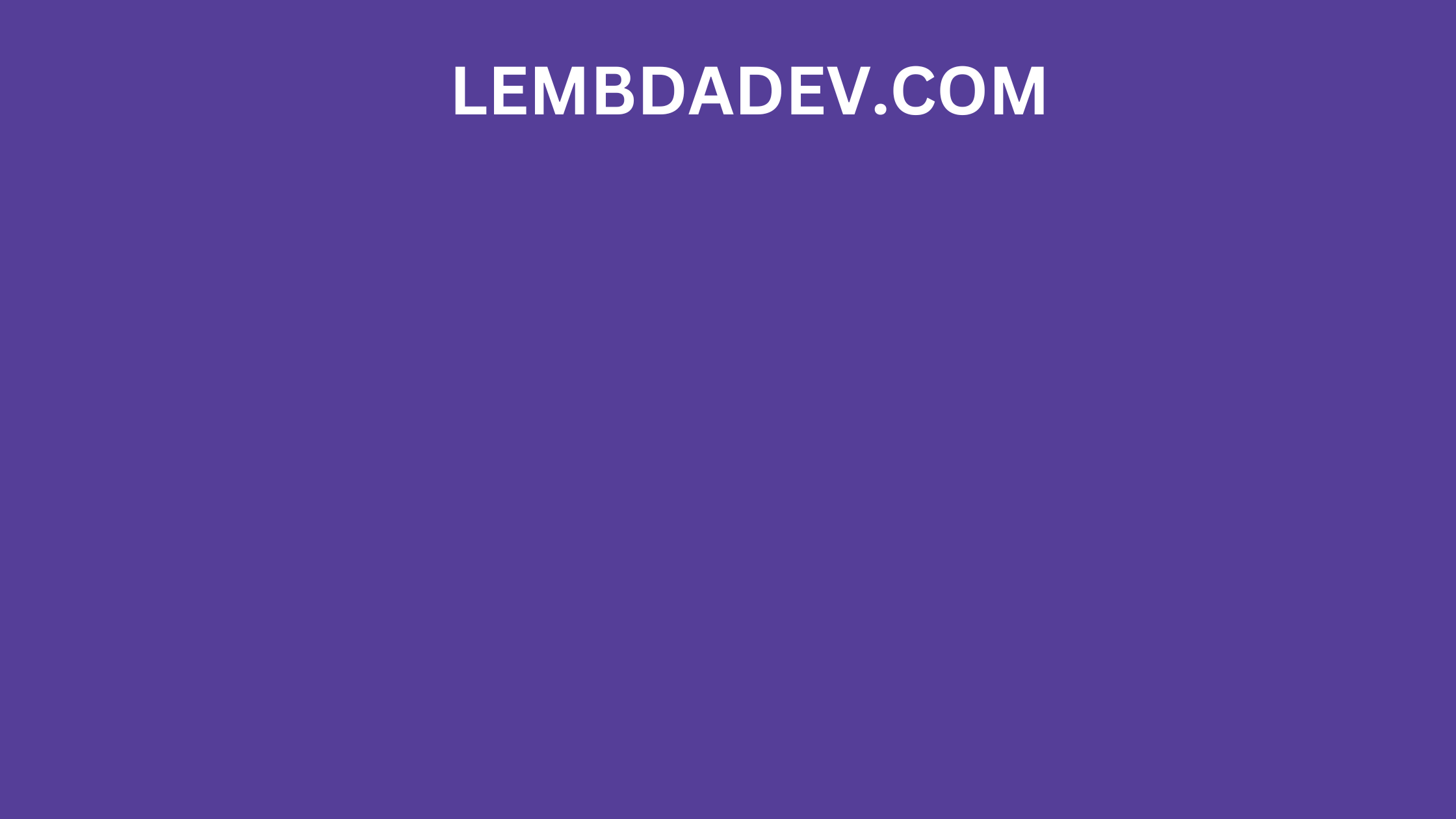
How to create a style tag using JavaScript?
How to create a style tag using JavaScript?
To create a style tag using JavaScript, you can use the following steps:
- Create a new element using the document.createElement() method.
- Set the element's tagName property to "style".
- Set the element's textContent property to the CSS code you want to add to the style tag.
- Append the element to the head of the document using the document.head.appendChild() method.
Example of how to create a style tag using JavaScript:
const styleElement = document.createElement("style");
styleElement.textContent = `
body {
color: red;
}
`;
document.head.appendChild(styleElement);
This code will create a new style tag and add it to the head of the document. The CSS code in the style tag will change the body text color to red.
You can also use the insertAdjacentHTML() method to create a style tag using JavaScript. The insertAdjacentHTML() method allows you to insert HTML code into the DOM at a specified position.
Example of how to create a style tag using the insertAdjacentHTML() method:
document.head.insertAdjacentHTML(
"beforeend",
`<style>body { color: red; }</style>`
);
This code will create a new style tag and add it to the end of the head element. The CSS code in the style tag will change the body text color to red.
Which method you use to create a style tag using JavaScript depends on your personal preference. The createElement() method is more explicit, but the insertAdjacentHTML() method is more concise.