- Published on
How to make HTTP requests with Axios in TypeScript?
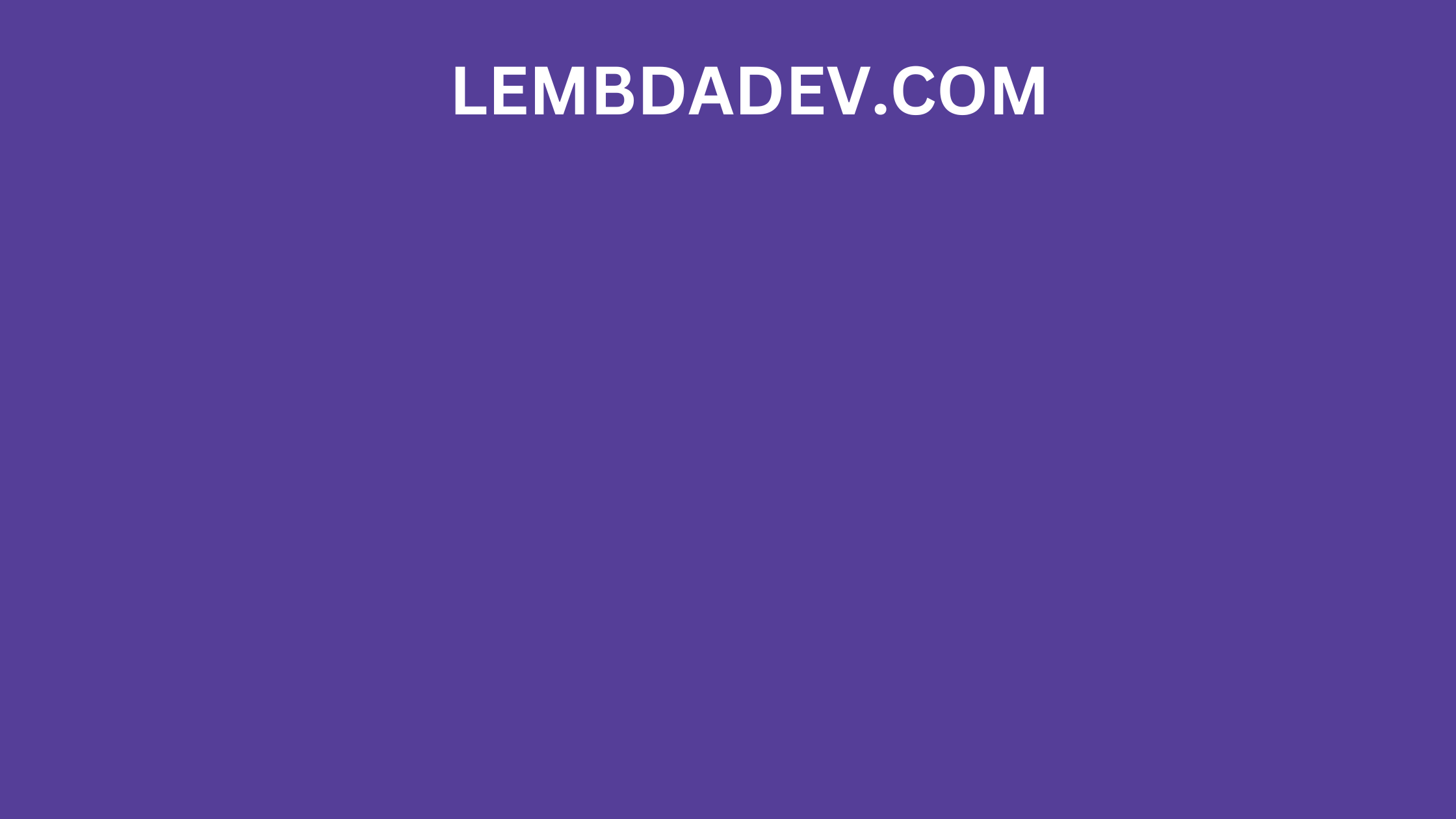
How to make HTTP requests with Axios in TypeScript?
How to make HTTP requests with Axios in TypeScript
Axios is a popular HTTP client library for JavaScript and TypeScript. It is easy to use and provides a powerful set of features, including:
- Support for all HTTP methods (GET, POST, PUT, DELETE, etc.)
- Automatic JSON serialization and deserialization
- Promise-based API for handling asynchronous requests
- Support for cancellation tokens
- Interceptors for handling common tasks such as authentication and error handling
How to use Axios in TypeScript
To use Axios in TypeScript, you first need to install it. You can do this using npm or Yarn:
npm install axios
yarn add axios
Once Axios is installed, you can import it into your TypeScript code:
import axios from "axios";
Now, you can start making HTTP requests using Axios. To make a GET request, simply call the axios.get()
method:
axios
.get("https://example.com/api/users")
.then((response) => {
// Handle the success response
})
.catch((error) => {
// Handle the error response
});
The axios.get()
method returns a Promise, so you can use async/await
to handle the response:
async function getUsers() {
const response = await axios.get("https://example.com/api/users");
return response.data;
}
The response.data
property contains the data that was returned from the API.
Making different HTTP requests with Axios
Axios can be used to make all kinds of HTTP requests, including GET, POST, PUT, DELETE, and more. To make a different type of HTTP request, simply call the corresponding Axios method:
axios.post()
to make a POST requestaxios.put()
to make a PUT requestaxios.delete()
to make a DELETE request
These methods all work in the same way as the axios.get()
method. They all return a Promise, and you can use async/await
to handle the response.
Here is an example of how to make a POST request to create a new user:
const user = {
name: "John Doe",
email: "john.doe@example.com",
};
axios
.post("https://example.com/api/users", user)
.then((response) => {
// Handle the success response
})
.catch((error) => {
// Handle the error response
});
The user
object is the data that will be sent to the API in the POST request body.
Using request and response types in Axios
Axios can be used with TypeScript to define types for the request and response data. This can help to prevent errors and make your code more robust.
To define a type for the request data, you can use a TypeScript interface:
interface User {
name: string;
email: string;
}
const user: User = {
name: "John Doe",
email: "john.doe@example.com",
};
Now, you can pass the user
object to the axios.post()
method with type safety:
axios
.post<User>("https://example.com/api/users", user)
.then((response) => {
// Handle the success response
})
.catch((error) => {
// Handle the error response
});
Axios will automatically serialize the user
object to JSON before sending it in the POST request body.
To define a type for the response data, you can also use a TypeScript interface:
interface UserResponse {
data: User;
}
Now, you can use the UserResponse
interface to type the response
variable in the then()
callback:
axios
.post<User>("https://example.com/api/users", user)
.then((response: UserResponse) => {
// Handle the success response
const user: User = response.data;
})
.catch((error) => {
// Handle the error response
});
This will ensure that the user
variable is always of type User
, even if the API returns an unexpected response.
Conclusion
Axios is a powerful and easy-to-use HTTP client library for JavaScript and TypeScript. It can be used to make all kinds of HTTP requests, including GET, POST, PUT, DELETE, and more.