- Published on
How to add Strings as Numbers in JavaScript?
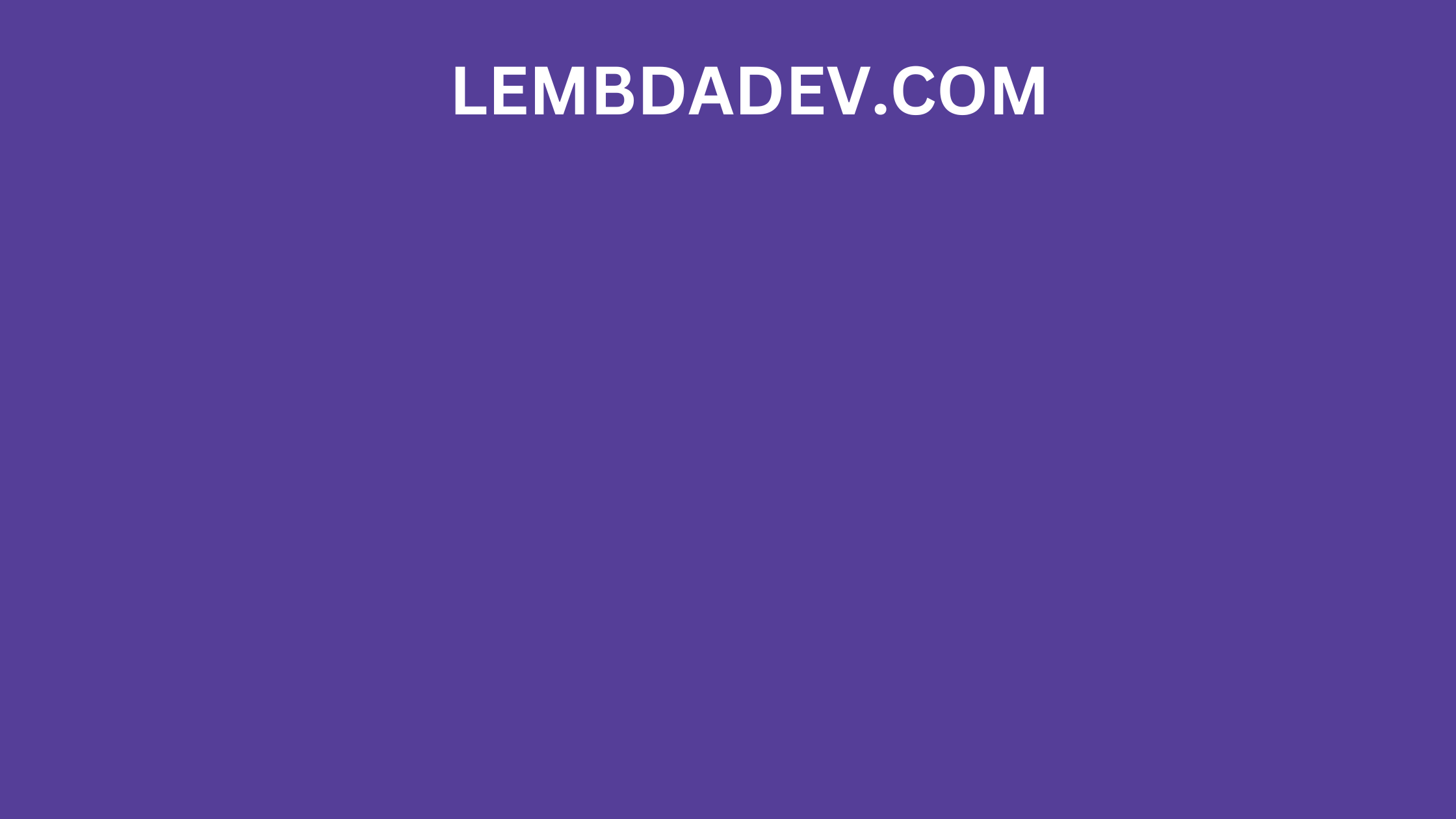
How to add Strings as Numbers in JavaScript?
How to add Strings as Numbers in JavaScript
JavaScript is a loosely typed language, which means that you can add strings and numbers together. However, when you add a string and a number, JavaScript will concatenate the two values instead of adding them together. This is because the
+
operator is used for both numeric addition and string concatenation.
To add strings as numbers in JavaScript, you need to first convert the strings to numbers. There are two ways to do this:
- Use the
Number()
constructor. TheNumber()
constructor converts a string to a number. For example, to convert the string"10"
to a number, you would use the following code:
const number = Number("10");
- Use the unary plus
(+)
operator. The unary plus(+)
operator can also be used to convert a string to a number. For example, the following code is equivalent to the code above:
const number = +"10";
Once you have converted the strings to numbers, you can add them together using the +
operator. For example, the following code will add the numbers 10 and 20 together and return the result, which is 30:
const number1 = Number("10");
const number2 = Number("20");
const sum = number1 + number2;
console.log(sum); // 30
Example:
// Add two strings as numbers using the Number() constructor
const sum1 = Number("10") + Number("20");
// Add two strings as numbers using the unary plus (+) operator
const sum2 = +"10" + +"20";
// Log both results to the console
console.log(sum1); // 30
console.log(sum2); // 30
Which method should I use?
The Number()
constructor and the unary plus (+)
operator are both valid ways to convert strings to numbers. However, the Number()
constructor is generally considered to be the better method. This is because it is more explicit and less likely to cause unexpected results.
Conclusion
Adding strings as numbers in JavaScript is a simple process. To do this, you first need to convert the strings to numbers using the Number()
constructor or the unary plus (+)
operator. Once you have converted the strings to numbers, you can add them together using the +
operator.